如何使用G.nodes(data=True)和G.edges(data=True)来获取这些信息。
时间: 2024-12-16 22:31:06 浏览: 16
在`networkx`库中,`G.nodes(data=True)` 和 `G.edges(data=True)` 是两个非常有用的函数,用于获取图中节点和边的详细信息。
- `G.nodes(data=True)`:这个函数返回一个字典,其中每个键是图中的节点,值是一个包含额外属性(如权重)的元组。例如:
```python
nodes_info = G.nodes(data=True)
for node, attrs in nodes_info.items():
print(f'Node {node}: {attrs}')
```
这将输出每个节点及其关联的所有属性,如边的权重。
- `G.edges(data=True)`:这个函数同样返回一个字典,但是这里的键是每条边,值是一个包含边的两端节点及额外属性的三元组。例如:
```python
edges_info = G.edges(data=True)
for edge, attrs in edges_info.items():
src, dst, attr_dict = attrs
print(f'Edge from {src} to {dst}: {attr_dict["weight"]}')
```
这里,`attr_dict`字典包含了边的全部信息,如“weight”。
使用这两个函数可以让你深入了解图结构以及边和节点之间的联系,特别是当你处理带权重的图时,如你之前创建的加权图。
相关问题
print(G1.nodes(data=True)) print(G1.edges(data=True))改成存储这两份数据
`print(G1.nodes(data=True))` 和 `print(G1.edges(data=True))` 分别用于打印图 `G1` 的所有节点及其属性,以及所有边及其相关的权重。如果你想要将这些数据存储起来而不是直接打印,可以使用pandas DataFrame结构,因为网络X图通常不会直接支持这样的直接序列化。
首先,你可以创建空的数据字典和边缘字典来储存节点和边的信息:
```python
node_data = {}
edge_data = {}
for node, attr_dict in G1.nodes(data=True):
node_data[node] = attr_dict
for edge, attr_dict in G1.edges(data=True):
edge_data[(edge[0], edge[1])] = attr_dict # 对于有向图,边的方向可能很重要,这里以元组形式存储
```
在这里,`node_data` 字典中的键是节点名,值是包含额外属性的字典;而`edge_data` 中的键是对的(源节点,目标节点),值是边的属性字典,包含旅行量等信息。
然后,你可以将这些字典转换成DataFrame,以便更方便地进行后续处理或存储:
```python
import pandas as pd
node_df = pd.DataFrame.from_dict(node_data, orient='index', columns=['Node', 'Additional attributes'])
edge_df = pd.DataFrame.from_dict(edge_data, orient='index', columns=['Source', 'Destination', 'Weight'])
# 存储至CSV文件
node_df.to_csv('nodes.csv')
edge_df.to_csv('edges.csv')
```
这样就分别保存了节点和边的数据到CSV文件中。
def minimum_cost_network(n,roads): G = nx.Graph() G.add_nodes_from(range(1,n+1)) G.add_weighted_edges_from(roads) min_tree = nx.minimum_spanning_tree(G) min_cost = sum(data["weight"] for u, v, data in min_tree.edges(data = True)) return min_cost
这是一个 Python 函数,它的作用是计算一个无向连通图的最小生成树的边权和。具体来说,它接受两个参数:一个整数 n 表示图中节点的数量,和一个包含 n-1 个元素的列表 roads,其中每个元素是一个三元组 (u, v, w),表示一条从节点 u 到节点 v 的有向边,边权为 w。函数使用 NetworkX 库构建了一个图对象 G,然后调用 NetworkX 库提供的 minimum_spanning_tree 函数计算最小生成树 min_tree,最后将 min_tree 的所有边的权值相加得到最小生成树的边权和 min_cost,然后将其返回。
阅读全文
相关推荐
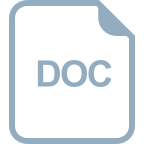
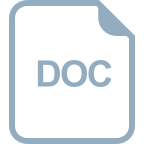
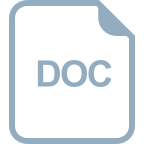




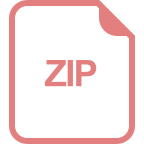
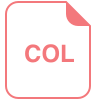
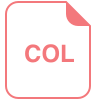
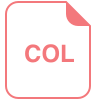
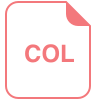




