C++ 中将几组字符串保存到CSV 中,后再分别读取其中某组字符串
时间: 2024-11-22 11:38:19 浏览: 5
在C++中,处理CSV(Comma-Separated Values)文件通常涉及到使用流(streams)和一些库函数,比如标准库中的`fstream`。以下是一个简单的步骤说明:
1. **写入CSV文件**:
首先,你需要包含必要的头文件,并定义一个函数来写入CSV数据。假设我们有两组字符串 `strGroup1` 和 `strGroup2`:
```cpp
#include <fstream>
#include <string>
void saveToCSV(const std::vector<std::string>& group1, const std::vector<std::string>& group2) {
std::ofstream file("data.csv");
if (file.is_open()) {
for (const auto& str : group1) {
file << str << ",";
}
file << "\n"; // 换行
for (const auto& str : group2) {
file << str << ",";
}
file.close();
} else {
std::cerr << "Unable to open file" << std::endl;
}
}
```
这段代码首先创建一个`std::ofstream`,然后遍历每组字符串,每项后加逗号分隔,最后换行。
2. **读取CSV文件**:
可以定义另一个函数来从文件中读取数据,例如:
```cpp
std::vector<std::string> readFromCSV() {
std::ifstream inputFile("data.csv");
std::vector<std::string> result;
if (inputFile.is_open()) {
std::string line;
while (getline(inputFile, line)) { // 逐行读取
size_t pos = 0;
std::string item;
while ((pos = line.find(",")) != std::string::npos) {
item = line.substr(0, pos);
result.push_back(item); // 将每一项添加到结果中
line.erase(0, pos + 1); // 移除已提取的部分
}
result.push_back(line); // 最后一行可能不包含逗号
}
inputFile.close();
} else {
std::cerr << "Unable to open file" << std::endl;
}
return result; // 返回所有字符串组成的向量
}
```
此函数会读取整个CSV文件,每次找到逗号就分割出一项字符串,并将其添加到结果列表中。
阅读全文
相关推荐
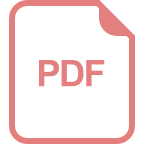
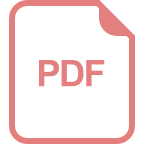
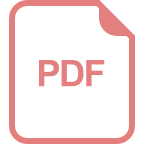
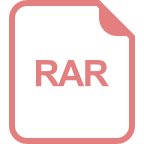
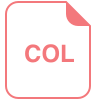
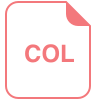
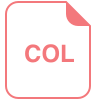
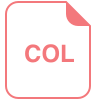
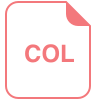
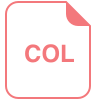
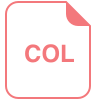



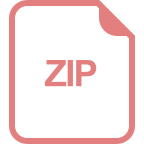
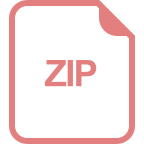
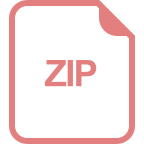