设计c++程序,使用结构定义数据:{学号(字符串,长度12),分数(整数),分数对应二进制(字符串,长度8)},先向csv文件写入16行不同的数据,然后从这个csv文件中将这16行数据读出屏幕打印出来(不含逗号,分割后的数据)。
时间: 2024-11-14 21:23:30 浏览: 21
在C++中,你可以使用`fstream`库来处理CSV文件操作,包括读取和写入数据。首先,你需要定义一个结构体(struct)来存储你要的数据:
```cpp
#include <iostream>
#include <fstream>
#include <string>
// 定义结构体
struct StudentData {
std::string studentID; // 学号
int score; // 分数
std::string binaryScore; // 分数的二进制形式(长度固定)
};
```
接下来,创建函数分别用于写入和读取CSV文件:
**写入CSV文件:**
```cpp
void writeToCSV(const std::vector<StudentData>& students, const std::string& filename) {
std::ofstream file(filename);
if (file.is_open()) {
for (const auto& data : students) {
file << data.studentID << "," << data.score << "," << data.binaryScore << "\n";
}
file.close();
std::cout << "Data written to CSV successfully.\n";
} else {
std::cerr << "Failed to open the file." << std::endl;
}
}
```
**从CSV文件读取并打印:**
```cpp
void readFromCSV(std::ifstream& file, std::vector<StudentData>& students) {
std::string line;
while (std::getline(file, line)) {
std::istringstream iss(line);
std::string id, strScore;
int score;
std::getline(iss, id, ',');
iss >> score;
std::stringstream binaryStream;
binaryStream << std::bitset<8>(score);
std::getline(binaryStream, strScore, ',');
students.push_back({id, score, strScore});
}
std::cout << "Data from CSV printed:\n";
for (const auto& data : students) {
std::cout << "Student ID: " << data.studentID << ", Score: " << data.score << ", Binary Score: " << data.binaryScore << '\n';
}
}
int main() {
// 创建学生数据
std::vector<StudentData> students = {
// 16行不同的数据...
};
// 写入CSV
writeToCSV(students, "students.csv");
// 读取CSV并打印
std::ifstream inputFile("students.csv");
readFromCSV(inputFile, students);
return 0;
}
```
请记住替换`students`变量里的16行数据,并运行`main()`函数来实现整个流程。
阅读全文
相关推荐
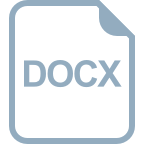
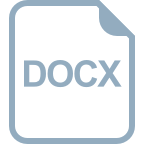
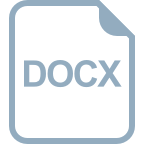

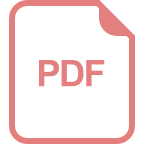
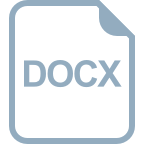
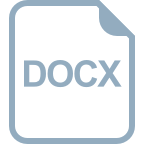
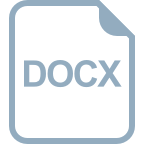
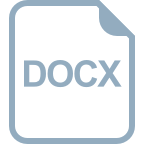
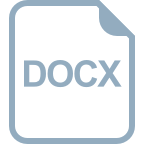
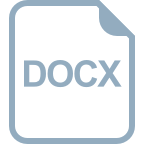
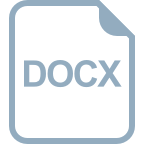
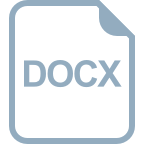
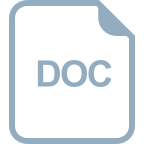
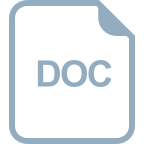
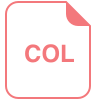
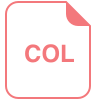
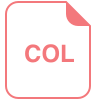