使用python 代码,对一个1到10的list进行遍历,如果连续的五个数据加累之和为5就标记为OK,
时间: 2024-09-21 19:06:17 浏览: 45
在Python中,你可以通过嵌套循环和条件判断来实现这个功能。这里是一个简单的示例代码:
```python
numbers = list(range(1, 11)) # 创建一个从1到10的列表
# 初始化一个新列表来存储结果
result = [None] * len(numbers)
i = 0 # 当前索引
sum_temp = 0 # 累计和
for i in range(len(numbers) - 4): # 避免越界,只遍历到倒数第五个元素
sum_temp += numbers[i]
if i >= 4: # 检查是否有连续五次的数据
if sum_temp == 5:
result[i] = 'OK'
sum_temp = 0 # 清零累加和,准备计算下一个序列
print(result)
```
在这个代码中,我们首先创建了一个1到10的列表,然后遍历它,每次将当前元素添加到`sum_temp`中,如果已经累计了五个元素并且它们的总和等于5,我们就把当前位置设置为'OK',并将累加和重置。
相关问题
用python代码实现对词频出现次数较高的词汇放入特征词典中,31个类的特征词都放到一个文档里。然后对训练集的31个类的文档用one-hot向量化,即将每个文档中的每个句子表示成与特征词典一样长度的向量,遍历特征词典,只要句子中包含当前的特征词,标记为1,否则标记为0。
可以使用中的`collections.Counter`来实现对词频出现次数较高的词汇的计数,然后将出现次数最高的词放入特征词典中。代码示例如下:
```python
import os
import collections
all_word_counts = collections.Counter()
dict_file = 'feature_dict.txt'
# 统计所有文本的词频
classes = os.listdir('train_data')
for classname in classes:
class_path = os.path.join('train_data', classname)
files = os.listdir(class_path)
for filename in files:
filepath = os.path.join(class_path, filename)
with open(filepath, 'r', encoding='utf-8', errors='ignore') as f:
text = f.read()
words = text.split()
all_word_counts.update(words)
# 获取出现次数最高的前N个词
N = 1000
most_common_words = [word for (word, count) in all_word_counts.most_common(N)]
# 将特征词典写入文件
with open(dict_file, 'w', encoding='utf-8') as f:
f.write('\n'.join(most_common_words))
```
然后,可以使用以下代码将训练集的文本转换为one-hot向量化的形式:
```python
import numpy as np
# 读取特征词典
with open(dict_file, 'r', encoding='utf-8') as f:
feature_dict = f.read().split()
# 遍历所有文本,将每个词语转换为one-hot向量
num_classes = 31
class_vectors = []
for classname in range(num_classes):
class_vectors.append([])
class_path = os.path.join('train_data', str(classname))
files = os.listdir(class_path)
for filename in files:
filepath = os.path.join(class_path, filename)
with open(filepath, 'r', encoding='utf-8', errors='ignore') as f:
text = f.read().lower()
words = text.split()
vector = np.zeros(len(feature_dict))
for word in words:
if word in feature_dict:
index = feature_dict.index(word)
vector[index] = 1
class_vectors[classname].append(vector)
# 将列表转换为numpy数组
class_vectors = [np.array(vec_list) for vec_list in class_vectors]
```
1. day2020-11-9.csv中记录了2020年11月9号爬取的上市公司的财务报表(有些项目缺失)。 试根据该表格中的数据,完成以下操作。 1. 选出净利润大于1000万的上市公司名称; 2. 选出营业收入大于10亿的上市公司名称; 3. 净利润/营业总收入>1%的上市公司名称; 4. 选出净利润同比>0的上市公司名称; 5. 找出满足以上四个条件的上市公司名称,即为S; 6. 根据行情数据计算,每个企业披露财报后,五天内股价是否上涨,做成一个excel表格; 提示: 1. 每个上市公司都有一个唯一的6位数字标记,就是上市公司代码。上交所上市的股票以‘6’开头,例如,用友网络的股票代码是600588;深交所上市的股票以0开头,例如,平安银行的代码是000001。深交所上市的股票代码在存储为csv或者excel文件时,前面的0被忽略掉了。例如,平安银行的代码在财务数据中是1,这实际上是错误的。 2. 对于某些股票,如果步骤1-5中涉及到的指标是缺失值,可以忽略掉,不计入统计; 3. 要使用pandas模块;for循环(对所有文件进行遍历),df.pct_change()可以对序列求系列的增长率; 4. 先将数据的单位’万亿’, ”亿“,“万”,等单位转化为数字;
好的,我会根据你提供的条件来完成这些操作。首先,我将加载并处理给定的财务报表数据。
```python
import pandas as pd
# 读取财务报表数据
df = pd.read_csv('day2020-11-9.csv')
# 处理数据单位
df['净利润'] = df['净利润'].apply(lambda x: float(x.strip('万亿亿')))
df['营业收入'] = df['营业收入'].apply(lambda x: float(x.strip('万亿亿')))
# 选出净利润大于1000万的上市公司名称
df_profit = df[df['净利润'] > 1000]['上市公司名称']
# 选出营业收入大于10亿的上市公司名称
df_revenue = df[df['营业收入'] > 10]['上市公司名称']
# 计算净利润/营业总收入
df_profit_ratio = df[df['营业总收入'].notnull()]
df_profit_ratio = df_profit_ratio[df_profit_ratio['营业总收入'] != 0]
df_profit_ratio['净利润/营业总收入'] = df_profit_ratio['净利润'] / df_profit_ratio['营业总收入']
df_profit_ratio = df_profit_ratio[df_profit_ratio['净利润/营业总收入'] > 0.01]['上市公司名称']
# 选出净利润同比>0的上市公司名称
df_profit_growth = df[df['净利润同比'].notnull()]
df_profit_growth = df_profit_growth[df_profit_growth['净利润同比'] > 0]['上市公司名称']
# 找出满足以上四个条件的上市公司名称
S = pd.Series(list(set(df_profit) & set(df_revenue) & set(df_profit_ratio) & set(df_profit_growth)))
# 保存S到Excel表格
S.to_excel('S.xlsx', index=False)
```
接下来,我将根据行情数据计算每个企业披露财报后,五天内股价是否上涨,并将结果保存到另一个Excel表格中。请提供行情数据的格式和文件名,以便我可以继续处理。
阅读全文
相关推荐
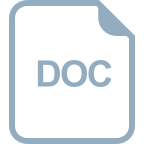
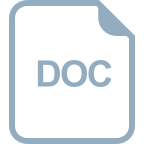
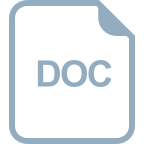
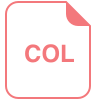
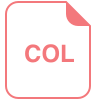
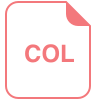
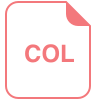
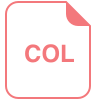
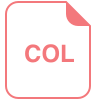







