fpgrowth代码
时间: 2023-09-15 19:18:33 浏览: 48
当然,我可以为您提供一个简单的FPGrowth算法的代码示例,以使用Python实现。下面是一个基本的实现:
```python
from collections import defaultdict
class FPNode:
def __init__(self, item, count, parent):
self.item = item
self.count = count
self.parent = parent
self.link = None
self.children = defaultdict()
def add_child(self, child):
if child.item not in self.children:
self.children[child.item] = child
class FPTree:
def __init__(self):
self.root = FPNode(None, 0, None)
self.header_table = defaultdict()
def add_transaction(self, transaction):
curr_node = self.root
for item in transaction:
if item in curr_node.children:
curr_node.children[item].count += 1
else:
new_node = FPNode(item, 1, curr_node)
curr_node.add_child(new_node)
if item in self.header_table:
self._update_header_table(item, new_node)
else:
self._insert_header_table(item, new_node)
curr_node = curr_node.children[item]
def _insert_header_table(self, item, node):
self.header_table[item] = [node, node]
def _update_header_table(self, item, node):
start_node = self.header_table[item][1]
while start_node.link is not None:
start_node = start_node.link
start_node.link = node
def get_frequency_items(self, min_support):
items = []
for item in self.header_table:
count = sum([n.count for n in self._get_paths(item)])
if count >= min_support:
items.append((item, count))
return items
def _get_paths(self, item):
start_node = self.header_table[item][0]
paths = []
while start_node is not None:
path = []
curr_node = start_node.parent
while curr_node.item is not None:
path.append(curr_node.item)
curr_node = curr_node.parent
if len(path) > 0:
paths.append((path[::-1], start_node.count))
start_node = start_node.link
return paths
def fp_growth(transactions, min_support):
tree = FPTree()
for transaction in transactions:
tree.add_transaction(transaction)
freq_items = tree.get_frequency_items(min_support)
if len(freq_items) == 0:
return []
frequent_patterns = []
for item, support in freq_items:
frequent_patterns.append(([item], support))
suffixes = _get_suffixes(tree, item)
sub_transactions = _get_sub_transactions(suffixes)
sub_patterns = fp_growth(sub_transactions, min_support)
for pattern in sub_patterns:
frequent_patterns.append(([item] + pattern[0], pattern[1]))
return frequent_patterns
def _get_suffixes(tree, item):
suffixes = []
start_node = tree.header_table[item][0]
while start_node is not None:
suffix = []
curr_node = start_node.parent
while curr_node.item is not None:
suffix.append(curr_node.item)
curr_node = curr_node.parent
if len(suffix) > 0:
suffixes.append((suffix[::-1], start_node.count))
start_node = start_node.link
return suffixes
def _get_sub_transactions(suffixes):
sub_transactions = []
for suffix, count in suffixes:
sub_transactions.extend([suffix] * count)
return sub_transactions
# 示例用法:
transactions = [['A', 'B', 'D'],
['B', 'D'],
['A', 'C', 'D', 'E'],
['A', 'D', 'E'],
['B', 'C', 'D']]
min_support = 3
frequent_patterns = fp_growth(transactions, min_support)
for pattern, support in frequent_patterns:
print(f"{pattern}: {support}")
```
这段代码实现了一个简单的FP-Growth算法,用于发现给定数据集中的频繁模式。它首先构建了一个FP树,然后通过递归遍历FP树来找到频繁模式。在示例中,我们使用一个简单的交易数据集进行演示,并设置最小支持度为3。输出将显示所有频繁模式及其支持度。
请注意,这只是FP-Growth算法的基本实现,并可能需要进一步的优化和调整以适应您的具体需求。
相关推荐
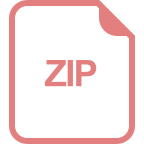














