BF及KMP算法完整字符串匹配程序;包括C语言代码详细解释及功能设计说明。
时间: 2024-11-09 21:18:33 浏览: 59
BF(Boyer-Moore Hunt)算法是一种高效的字符串搜索算法,而KMP(Knuth-Morris-Pratt)算法则是另一种常用的字符串匹配算法。它们都是在文本串(主串)中查找目标串的技术。
**BF算法(Bad Character Rule):**
BF算法利用了字符坏序的思想,当遇到不匹配的字符时,它会直接跳过主串中大于当前目标字符的最大偏移量。这个算法的优点是在某些情况下可以达到线性的速度,但它需要额外的空间存储坏字符信息,对于大数据处理可能会有性能损失。
**KMP算法(Knuth-Morris-Preparatory Algorithm):**
KMP算法通过构建部分匹配表(Partial Match Table,PMT),预先计算出模式串的失配位置,当模式串和主串发生匹配失败时,可以根据PMT直接跳过错误的位置,避免了许多不必要的比较。KMP算法不需要额外空间,时间复杂度也是O(n)。
以下是C语言的基本实现:
```c
#include <stdio.h>
#include <string.h>
// 定义部分匹配表
void compute_pmt(char pattern[], int pm[])
{
int len = strlen(pattern);
pm[0] = -1;
for (int i = 1; i < len; ++i)
pm[i] = pm[i - 1];
for (int j = 2, k = 0; j < len; ++j)
{
while (k > 0 && pattern[k] != pattern[j])
k = pm[k - 1];
if (pattern[k] == pattern[j])
++k;
pm[j] = k;
}
}
// KMP字符串匹配函数
int kmp_search(char text[], char pattern[])
{
int len_text = strlen(text);
int len_pattern = strlen(pattern);
int i = 0, j = 0;
compute_pmt(pattern, pattern_pm);
while (i < len_text && j < len_pattern)
{
if (text[i] == pattern[j])
{
i++;
j++;
}
else if (j > 0)
j = pattern_pm[j - 1];
else
i++;
}
return j == len_pattern ? i - j : -1;
}
int main()
{
char text[] = "ABABCABABC";
char pattern[] = "ABC";
int index = kmp_search(text, pattern);
if (index != -1)
printf("Pattern found at index %d\n", index);
else
printf("Pattern not found.\n");
return 0;
}
```
在这个例子中,`compute_pmt`函数用于构建部分匹配表,`kmp_search`函数则使用该表进行字符串匹配。如果找到匹配,则返回开始索引,否则返回-1表示未找到。
阅读全文
相关推荐
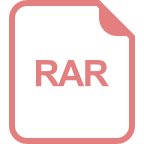
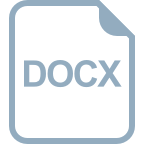

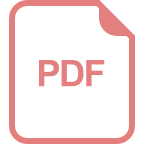
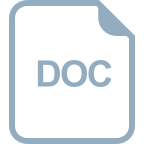
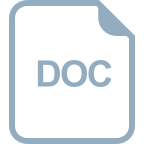
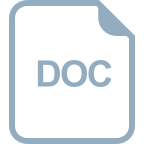









