python多线程的实现方式
时间: 2024-04-23 20:28:11 浏览: 24
Python中实现多线程有多种方式,以下是其中两种常用的方式:
1. 使用`threading`模块:`threading`模块提供了Thread类,可以派生出自定义的线程类。创建线程的方式有两种:继承Thread类和直接实例化Thread类。
a) 继承Thread类:
```python
import threading
class MyThread(threading.Thread):
def run(self):
# 线程执行的代码
# 创建并启动线程
thread = MyThread()
thread.start()
```
b) 直接实例化Thread类:
```python
import threading
def my_function():
# 线程执行的代码
# 创建并启动线程
thread = threading.Thread(target=my_function)
thread.start()
```
2. 使用`concurrent.futures`模块:`concurrent.futures`模块提供了ThreadPoolExecutor和ProcessPoolExecutor两个类,可以方便地实现线程池和进程池。
a) 线程池示例:
```python
from concurrent.futures import ThreadPoolExecutor
def my_function():
# 线程执行的代码
# 创建线程池
with ThreadPoolExecutor() as executor:
# 提交任务给线程池
future = executor.submit(my_function)
# 获取任务的结果
result = future.result()
```
b) 进程池示例:
```python
from concurrent.futures import ProcessPoolExecutor
def my_function():
# 进程执行的代码
# 创建进程池
with ProcessPoolExecutor() as executor:
# 提交任务给进程池
future = executor.submit(my_function)
# 获取任务的结果
result = future.result()
```
这些是Python中实现多线程的两种常用方式,具体选择哪种方式取决于你的需求和场景。
相关推荐
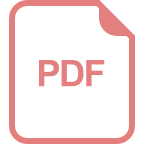
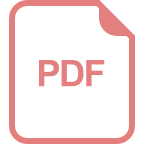
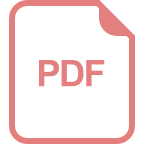














