用c语言写出进程通信方式的程序
时间: 2024-10-09 08:01:25 浏览: 17
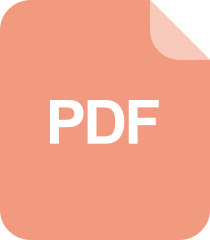
C语言实现进程间通信原理解析
在C语言中,有两种常见的进程间通信(IPC,Inter-Process Communication)方式:管道(pipe)和消息队列(message queue)。这里分别给出两个简单示例:
1. **管道通信**:
```c
#include <stdio.h>
#include <unistd.h>
#define BUFFER_SIZE 10
void parent(int pipe_fd) {
char input[BUFFER_SIZE];
printf("Parent: Please enter a message: ");
fgets(input, sizeof(input), stdin);
write(pipe_fd, input, strlen(input));
}
void child(int pipe_fd) {
char received[BUFFER_SIZE];
read(pipe_fd, received, BUFFER_SIZE);
printf("Child: Parent said: %s", received);
}
int main() {
int parent_pipe[2], child_pipe[2];
if (pipe(parent_pipe) == -1 || pipe(child_pipe) == -1) {
perror("Pipe creation failed");
return 1;
}
pid_t pid = fork();
if (pid == 0) { // Child process
close(parent_pipe[0]); // Close unused end of parent's pipe
child(child_pipe[1]);
close(child_pipe[1]);
} else { // Parent process
close(child_pipe[1]); // Close unused end of child's pipe
parent(parent_pipe[1]);
close(parent_pipe[1]);
}
return 0;
}
```
在这个例子中,父进程通过`write`将消息写入管道,子进程通过`read`读取并显示。
2. **消息队列通信**:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/mqueue.h>
mqd_t msg_queue;
void producer(char *msg) {
struct msqid_ds mds;
if (mq_open("/my_message_queue", O_CREAT | O_WRONLY, 0644, &mds) == -1) {
perror("Message queue open failed");
exit(1);
}
mq_send(msg_queue, msg, strlen(msg) + 1, 0); // Send message
mq_close(msg_queue);
}
void consumer(void) {
char buffer[100];
struct msqid_ds mds;
if (mq_open("/my_message_queue", O_RDONLY, 0, &mds) != 0) {
perror("Message queue open failed");
exit(1);
}
while (mq_receive(msg_queue, buffer, sizeof(buffer), NULL) > 0) {
printf("Consumer: Received message: %s\n", buffer);
}
mq_close(msg_queue);
}
int main() {
msg_queue = mq_open("/my_message_queue", O_CREAT | O_RDWR, 0644, NULL);
if (msg_queue == NULL) {
perror("Message queue creation failed");
exit(1);
}
// Producer and consumer tasks can be separated here or run in parallel.
// ...
return 0;
}
```
这个示例展示了如何创建和使用消息队列,生产者往队列中发送消息,消费者从队列中接收并处理。
阅读全文
相关推荐
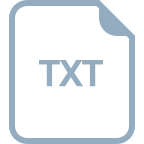
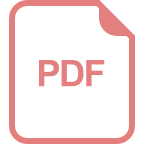



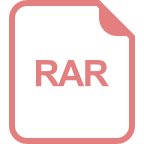
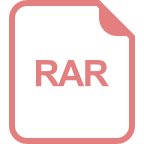
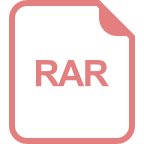
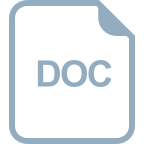
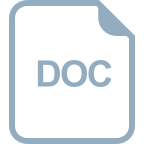
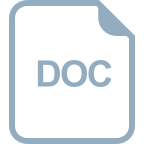
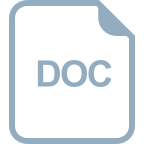





