nn.Module的forward什么时候调用
时间: 2024-09-14 19:08:15 浏览: 52
`nn.Module` 是 PyTorch 中所有神经网络模块的基类。`forward` 方法是在你创建了一个继承自 `nn.Module` 的子类并实现了这个方法之后,当你使用这个子类创建模型实例,并对数据调用模型实例时自动调用的。具体来说,当你执行模型实例化并传入输入数据时,如 `output = model(input_data)`,PyTorch 会自动调用 `forward` 方法,并将 `input_data` 作为参数传递给该方法。
以下是一段简化的代码示例来说明:
```python
import torch.nn as nn
class SimpleNet(nn.Module):
def __init__(self):
super(SimpleNet, self).__init__()
# 定义网络层等
def forward(self, x):
# 前向传播逻辑
return x + 1 # 假设的返回结果
# 实例化模型
model = SimpleNet()
# 调用模型进行前向传播
output = model(input_data)
```
在上面的例子中,当 `model(input_data)` 被调用时,`SimpleNet` 类中的 `forward` 方法会被自动执行。
相关问题
nn.Module的forward函数
nn.Module的forward函数是PyTorch框架中定义神经网络模块时必须要实现的一个方法。在继承nn.Module类创建一个新的网络模块类时,你需要定义一个forward函数来描述网络的前向传播过程。前向传播是指输入数据经过网络各层处理直到输出的过程。
例如,如果你创建了一个简单的全连接层网络,你可能会这样定义forward方法:
```python
import torch.nn as nn
class SimpleNet(nn.Module):
def __init__(self):
super(SimpleNet, self).__init__()
self.fc1 = nn.Linear(in_features=10, out_features=20)
self.fc2 = nn.Linear(in_features=20, out_features=1)
def forward(self, x):
x = self.fc1(x)
x = self.fc2(x)
return x
```
在这个例子中,forward方法接收输入数据x,通过两个全连接层fc1和fc2进行处理,并返回最终的输出。注意,forward方法定义了数据如何在网络中流动,而不需要显式调用反向传播函数或者更新权重,这些工作都是由PyTorch的优化器(如torch.optim.SGD)自动完成的。
nn.Module.register_forward_hook()如何使用
nn.Module.register_forward_hook()方法可以用来注册一个forward hook,用于在神经网络前向传递时执行一些操作,例如打印中间结果或提取特定层的表示。该方法的使用示例如下:
```python
import torch.nn as nn
def forward_hook(module, input, output):
# 在神经网络前向传递时执行的操作
pass
model = nn.Sequential(
nn.Linear(10, 20),
nn.ReLU(),
nn.Linear(20, 30),
nn.ReLU(),
nn.Linear(30, 10),
nn.Softmax(dim=1)
)
# 注册一个forward hook
hook_handle = model[2].register_forward_hook(forward_hook)
# 在一个batch的数据上进行前向传递
input = torch.randn(32, 10)
output = model(input)
# 移除forward hook
hook_handle.remove()
```
在上述示例中,我们注册了一个forward hook到模型的第三层Linear层上。在前向传递时,每当数据通过这一层时,forward_hook函数都会被调用。同时,我们可以通过hook_handle来随时移除该forward hook。
阅读全文
相关推荐
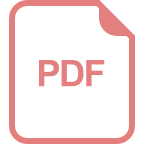
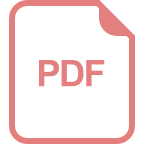
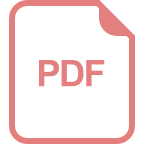













