建立一个汽车Auto类,包括轮胎个数、汽车颜色、车身重量、速度等成员变量,并通过不同的构造方法创建实例。至少要求:汽车能够加速、减速、停车。再定义一个小汽车类Car,继承 Auto,并添加空调、CD等成员变量,覆盖加速、减速的方法。代码及详细注释
时间: 2024-12-18 12:24:09 浏览: 13
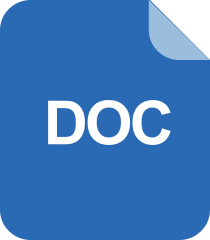
Java设计一个银行帐户类(继承与多态)

在Java中,我们可以建立一个名为`Auto`的基础汽车类和一个小汽车子类`Car`来满足这个需求。以下是详细的代码示例:
```java
// 定义基础汽车类Auto
public class Auto {
private int tireCount;
private String color;
private double weight;
private double speed;
// 默认构造函数
public Auto(int tireCount, String color, double weight) {
this.tireCount = tireCount;
this.color = color;
this.weight = weight;
this.speed = 0; // 初始化速度为0
}
// 具体构造函数,提供速度参数
public Auto(int tireCount, String color, double weight, double initialSpeed) {
this(tireCount, color, weight);
this.speed = initialSpeed;
}
// 加速方法,增加当前速度
public void accelerate(double acceleration) {
if (acceleration > 0) {
this.speed += acceleration;
} else {
System.out.println("Invalid acceleration.");
}
}
// 减速方法,减小当前速度
public void decelerate(double deceleration) {
if (deceleration > 0) {
this.speed -= deceleration;
if (this.speed < 0) {
this.speed = 0;
}
} else {
System.out.println("Invalid deceleration.");
}
}
// 停车方法,设置速度为0
public void stop() {
this.speed = 0;
}
// 获取类信息
@Override
public String toString() {
return "Auto [tireCount=" + tireCount + ", color=" + color + ", weight=" + weight + ", speed=" + speed + "]";
}
}
// 小汽车子类Car继承自Auto
public class Car extends Auto {
private boolean hasAirConditioner;
private boolean hasCDPlayer;
// 构造函数,引入新的特性
public Car(int tireCount, String color, double weight, double initialSpeed, boolean airConditioner, boolean cdPlayer) {
super(tireCount, color, weight, initialSpeed);
this.hasAirConditioner = airConditioner;
this.hasCDPlayer = cdPlayer;
}
// 重写加速方法,增加特色描述
@Override
public void accelerate(double acceleration) {
super.accelerate(acceleration);
if (hasAirConditioner && this.speed >= 60) {
System.out.println("Air conditioner turned on as speed exceeds 60 km/h.");
}
}
// 重写减速方法,增加特色描述
@Override
public void decelerate(double deceleration) {
super.decelerate(deceleration);
if (hasCDPlayer && this.speed <= 0) {
System.out.println("CD player starts playing when car stops.");
}
}
// 获取类信息
@Override
public String toString() {
return "Car [" +
"super=" + super.toString() +
", hasAirConditioner=" + hasAirConditioner +
", hasCDPlayer=" + hasCDPlayer +
"]";
}
}
```
在这个例子中,`Car`类继承了`Auto`类,添加了空调和CD播放机的成员变量,并覆盖了加速和减速方法,增加了额外的行为描述。
阅读全文
相关推荐
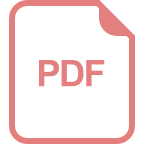










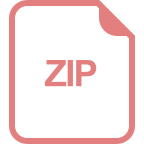