建立一个汽车 Auto 类 包括轮胎个数 汽车颜色 车身重量、速度等成员变量。并通过不同的构造方法创建实例。至少要求 汽车能够加速 减速 停车。再定义一个小汽车类 Car 继承 Auto 并添加空调、CD等成员变量 覆盖加速 减速的方法
时间: 2024-12-17 18:43:11 浏览: 4
在Java中,我们可以建立一个`Auto`类来描述汽车的基本属性,然后通过继承创建更具体的子类`Car`。以下是基本的设计:
```java
// 定义一个Auto类
public class Auto {
private int tires;
private String color;
private double weight;
private double speed;
// 默认构造方法
public Auto(int tires, String color, double weight) {
this.tires = tires;
this.color = color;
this.weight = weight;
this.speed = 0;
}
// 提供加速方法
public void accelerate(double increase) {
if (increase > 0) {
this.speed += increase;
} else {
throw new IllegalArgumentException("Speed can only be increased.");
}
System.out.println("Accelerating by " + increase + ", current speed is now " + this.speed);
}
// 提供减速方法
public void decelerate(double decrease) {
if (decrease > 0 && speed - decrease >= 0) {
this.speed -= decrease;
} else {
throw new IllegalArgumentException("Invalid deceleration amount.");
}
System.out.println("Decelerating by " + decrease + ", new speed is " + this.speed);
}
// 停车方法
public void stop() {
this.speed = 0;
System.out.println("Stopping the car.");
}
}
// 定义小汽车Car类,继承自Auto并添加额外特性
public class Car extends Auto {
private boolean hasAirConditioner;
private boolean hasCDPlayer;
// 添加特定于Car的构造方法
public Car(int tires, String color, double weight, boolean airConditioner, boolean cdPlayer) {
super(tires, color, weight);
this.hasAirConditioner = airConditioner;
this.hasCDPlayer = cdPlayer;
}
// 覆盖加速方法,这里可以添加更多功能如检查油量等
@Override
public void accelerate(double increase) {
super.accelerate(increase);
// 添加额外功能...
}
// 覆盖减速方法,同样可以添加额外控制
@Override
public void decelerate(double decrease) {
super.decelerate(decrease);
// 添加额外控制...
}
}
```
阅读全文
相关推荐









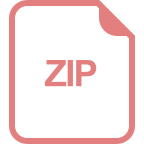
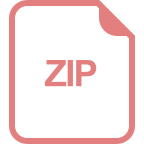