vector容器遍历输出
时间: 2024-09-09 17:17:37 浏览: 27
在C++中,`vector`是STL(Standard Template Library)的一个容器类,它是一个动态数组,可以存储任意类型的元素,并且能够自动管理内存。遍历并输出`vector`容器中的元素是一个常见的操作,可以通过多种方式实现,以下是一些常见的遍历输出方法:
1. 使用范围for循环(Range-based for loop):
```cpp
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> v = {1, 2, 3, 4, 5};
for (int i : v) {
cout << i << " ";
}
cout << endl;
return 0;
}
```
2. 使用迭代器(Iterator):
```cpp
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> v = {1, 2, 3, 4, 5};
for (auto it = v.begin(); it != v.end(); ++it) {
cout << *it << " ";
}
cout << endl;
return 0;
}
```
3. 使用下标访问(Index Access):
```cpp
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> v = {1, 2, 3, 4, 5};
for (size_t i = 0; i < v.size(); ++i) {
cout << v[i] << " ";
}
cout << endl;
return 0;
}
```
4. 使用基于指针的数组遍历方式(C风格):
```cpp
#include <iostream>
#include <vector>
using namespace std;
int main() {
vector<int> v = {1, 2, 3, 4, 5};
for (int* p = v.data(); p != v.data() + v.size(); ++p) {
cout << *p << " ";
}
cout << endl;
return 0;
}
```
以上方法中,推荐使用范围for循环和迭代器的方式,因为它们更为现代和安全,且迭代器方式在遍历STL容器时能够提供更多的灵活性。
相关推荐
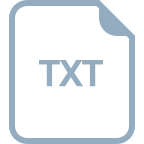
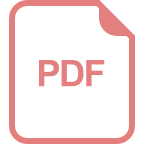
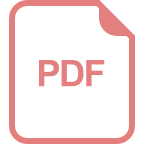
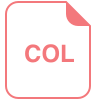
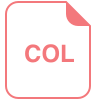
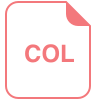
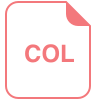
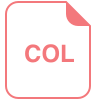









