delphi提取memo字符串中的所有数字
时间: 2024-09-24 14:28:58 浏览: 43
在Delphi中,你可以使用正则表达式库(`System.RegularExpressions`)来从Memo组件的文本中提取所有的数字。以下是一个简单的示例:
```delphi
uses
System.RegularExpressions;
function ExtractNumbers(const MemoText: string): TStringDynArray;
var
regex: TRegEx;
matches: TArray<string>;
begin
regex := TRegEx.Create('[-+]?\d+\.?\d*'); // 正则表达式匹配整数和小数
matches := regex.Execute(MemoText); // 执行搜索
Result := SetLength(TStringDynArray.Create, Matches.Count);
for I := 0 to High(matches) do
begin
Result[I] := matches[I].Value; // 将每个匹配的数字添加到数组中
end;
end;
```
这个函数会返回一个字符串动态数组,包含了Memo中所有的数字。
相关问题
delphi编程提取memo组件中字符串的所有数字
在Delphi编程中,如果你想从Memo组件中的字符串中提取所有的数字,你可以使用正则表达式(Regular Expressions)。Delphi提供了一个名为`FMTSCANF`的函数或者你可以使用第三方库如`RegEx`来完成这个任务。这里是一个简单的示例:
```delphi
uses
System.SysUtils, System.RegularExpressions;
function ExtractNumbersFromMemo(MemoText: string): TStringDynArray;
var
Numbers: TArray<string>;
RE: TRegEx;
begin
// 创建正则表达式对象,匹配所有数字
RE := TRegEx.Create('[-+]?[0-9]+(\.[0-9]+)?');
// 使用正则表达式查找所有匹配项
Numbers := RE.Execute(MemoText);
// 将结果转换为动态数组并返回
result := Numbers.Items;
end;
// 示例用法
var
MemoContent: string;
NumberList: TStringDynArray;
begin
MemoContent := Memo1.Text; // 假设Memo1是你想要提取的Memo组件
NumberList := ExtractNumbersFromMemo(MemoContent);
for I := 0 to High(NumberList) do
Writeln('提取到的数字:', NumberList[I]);
delphi编程提取memo组件中的所有数字
Delphi编程语言中的Memo组件通常是一个富文本编辑框,可以存储包括文字、图像等多种格式的数据。如果你想从Memo中提取所有的数字,你可以通过正则表达式或者遍历字符串的方式来实现。以下是基本步骤:
1. **使用正则表达式**:
使用`FindAll()`函数和一个正则表达式模式来查找所有数字。例如,你可以用`\d+`匹配一个或多个连续的数字字符。
```pascal
var
MemoContent: string;
Matches: TMatchCollection;
MemoContent := Memo1.Text; // 获取Memo组件的内容
var_pattern := '(\d+)'; // 正则表达式模式匹配数字
Matches := TRegEx.FindAll(MemoContent, var_pattern);
for Match in Matches do
begin
ShowMessage('Found number: ' + Match[0]); // 显示找到的每个数字
end;
```
2. **遍历字符串**:
另一种方法是从文本中逐字符检查,如果遇到数字字符,则记录下来。
```pascal
var
StartPos, EndPos: Integer;
FoundNum: Boolean = False;
StartPos := 0;
while not EndOfWord(StartPos) do // 查找下一个单词开始位置
begin
if IsDigit(Memo1.CharAt(StartPos)) then // 如果当前字符是数字
begin
FoundNum := True;
while IsDigit(Memo1.CharAt(StartPos)) and not EndOfWord(StartPos) do // 遍历到非数字字符为止
Inc(StartPos);
// 提取并处理数字
EndPos := StartPos - 1; // 减一是因为EndOfWord会包含最后一个数字字符
ExtractWord(Memo1.Text, MemoContent, StartPos, EndPos);
// 然后这里你可以解析或显示这个数字
end
else
begin
FoundNum := False;
end;
Inc(StartPos);
end;
```
阅读全文
相关推荐
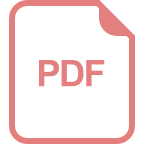
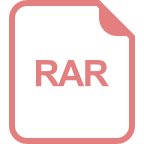
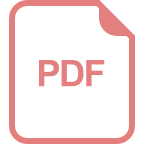
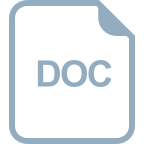
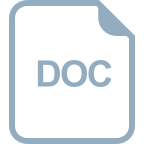
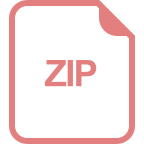
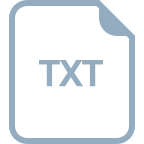
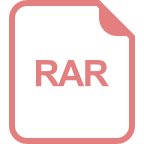
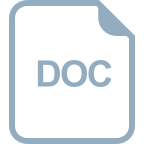
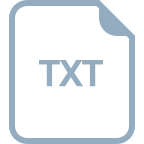
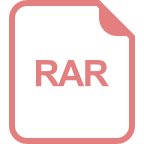
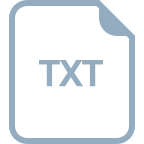
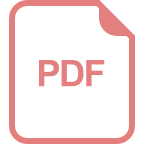
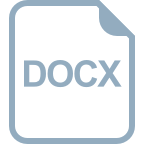