elasticsearch在python中的常见用法
时间: 2024-09-26 17:05:01 浏览: 34
Elasticsearch是一个开源的全文搜索和分析引擎,常用于构建实时、高性能的搜索引擎。在Python中,我们通常使用官方推荐的客户端库`elasticsearch`来进行操作。以下是它的一些常见用法:
1. **安装及导入**:
```shell
pip install elasticsearch
```
在Python中导入:
```python
from elasticsearch import Elasticsearch
```
2. **连接到Elasticsearch集群**:
```python
es = Elasticsearch([{'host': 'localhost', 'port': 9200}])
```
3. **创建索引**:
```python
index_name = "test_index"
mapping = { ... } # 定义映射结构
es.indices.create(index=index_name, body=mapping)
```
4. **文档插入**:
```python
doc = {"title": "My Document", "content": "This is a sample"}
res = es.index(index=index_name, id=1, body=doc)
```
5. **查询数据**:
```python
query = {
"query": {
"match": {"title": "Document"}
}
}
response = es.search(index=index_name, body=query)
hits = response['hits']['hits']
```
6. **更新和删除文档**:
```python
res = es.update(index=index_name, id=1, body={"doc": {"content": "Updated content"}})
res = es.delete(index=index_name, id=1)
```
7. **监控和管理**:
使用`elasticsearch.helpers`模块进行批量操作和分页查询,如bulk API 和 scroll API。
8. **异常处理**:
```python
try:
response = es.get(index=index_name, id=1)
except Exception as e:
print(f"Error: {e}")
```
阅读全文
相关推荐
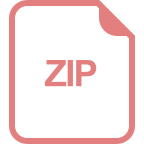
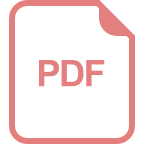
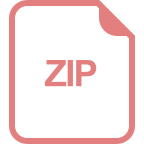
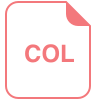
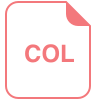
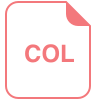
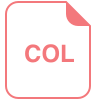
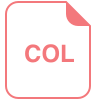
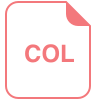
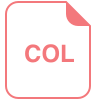
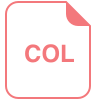
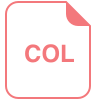
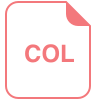
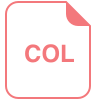
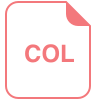
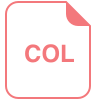
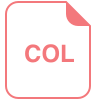
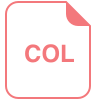
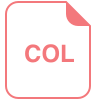