ubuntu c++串口读写
时间: 2024-10-11 08:00:17 浏览: 41
Ubuntu下的C++通过串口进行读写通常涉及到`iostream`库、`termios`库以及`fcntl.h`等头文件,因为Linux系统提供了`/dev/ttyS0`(或者其他序列端口名称)这样的设备来进行串口通信。以下是基本步骤:
1. 包含必要的头文件:
```cpp
#include <iostream>
#include <fstream>
#include <unistd.h> // for usleep() for delaying reads/writes
#include <sys/ioctl.h>
#include <termios.h>
```
2. 打开串口设备:
```cpp
int fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY); // 根据实际设备替换
if (fd == -1) {
std::cerr << "Failed to open serial port" << std::endl;
return -1;
}
```
3. 设置串口配置(比如波特率、数据位、停止位、校验位):
```cpp
struct termios options;
tcgetattr(fd, &options);
cfsetispeed(&options, B9600); // 设定波特率为9600bps,其他设置根据需求调整
cfsetospeed(&options, B9600);
tcflush(fd, TCIFLUSH); // 清空输入缓冲区
```
4. 发送数据:
```cpp
std::string data_to_send = "Hello from C++";
write(fd, data_to_send.c_str(), data_to_send.size());
```
5. 接收数据:
```cpp
char buffer[128];
size_t bytes_received = read(fd, buffer, sizeof(buffer));
if (bytes_received > 0) {
std::cout << "Received: " << buffer << std::endl;
} else if (bytes_received == 0) {
std::cerr << "End of file reached" << std::endl;
}
```
6. 关闭串口:
```cpp
close(fd);
```
阅读全文
相关推荐
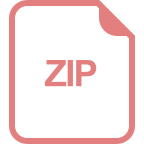

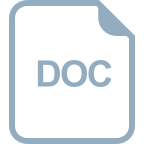

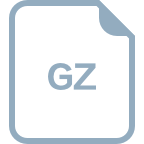
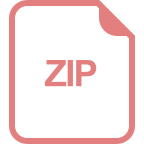
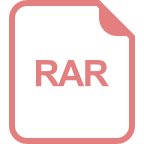
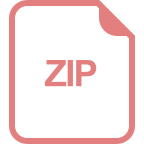
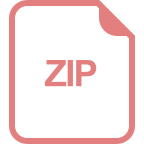
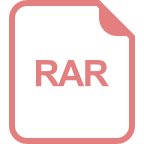
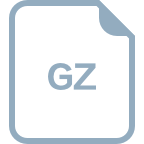
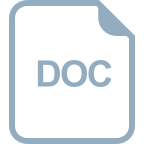
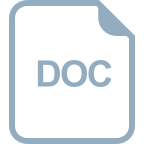

