python华容道广度
时间: 2023-10-21 07:28:43 浏览: 46
华容道是一种经典的拼图游戏,目标是通过移动方块来达到特定的布局。解决华容道可以采用广度优先搜索算法(BFS)。下面是一个示例代码:
```python
from collections import deque
# 定义华容道游戏的初始状态和目标状态
initial_state = [[1, 2, 3],
[4, 0, 6],
[7, 5, 8]]
target_state = [[1, 2, 3],
[4, 5, 6],
[7, 8, 0]]
# 定义移动的四个方向:上、下、左、右
directions = [(0, -1), (0, 1), (-1, 0), (1, 0)]
movements = ['上', '下', '左', '右']
# 使用广度优先搜索算法解决华容道
def solve_puzzle(initial_state, target_state):
visited = set()
queue = deque([(initial_state, [])])
while queue:
current_state, path = queue.popleft()
if current_state == target_state:
return path
visited.add(tuple(map(tuple, current_state)))
# 查找空格位置
zero_row, zero_col = find_zero(current_state)
for direction, movement in zip(directions, movements):
new_row = zero_row + direction[0]
new_col = zero_col + direction[1]
if 0 <= new_row < 3 and 0 <= new_col < 3:
new_state = [list(row) for row in current_state]
new_state[zero_row][zero_col], new_state[new_row][new_col] = new_state[new_row][new_col], new_state[zero_row][zero_col]
if tuple(map(tuple, new_state)) not in visited:
queue.append((new_state, path + [movement]))
visited.add(tuple(map(tuple, new_state)))
return None
# 找到空格的位置
def find_zero(state):
for i in range(3):
for j in range(3):
if state[i][j] == 0:
return i, j
# 测试解决华容道
solution = solve_puzzle(initial_state, target_state)
if solution:
print("移动步骤:")
for step, movement in enumerate(solution):
print(f"第{step+1}步: {movement}")
else:
print("无解")
```
这段代码使用了广度优先搜索算法(BFS)来解决华容道问题。首先定义了游戏的初始状态和目标状态,然后使用一个队列来进行广度优先搜索。在搜索过程中,每次从队列中取出当前状态,判断是否为目标状态,如果是,则返回移动的路径;否则,依次尝试四个方向的移动(上、下、左、右),并将移动后的状态加入队列。为了避免重复搜索相同的状态,使用一个集合来记录已经访问过的状态。最后,输出移动的步骤。
以上是一个简单示例,你可以根据实际情况进行修改和扩展。
相关推荐
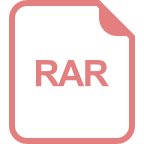
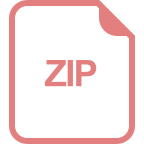
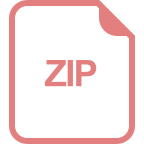














