python解华容道问题的程序
时间: 2024-03-20 08:38:54 浏览: 19
下面是一个简单的Python程序,可以解决华容道问题。程序使用广度优先搜索算法,遍历所有可能的移动序列,直到找到解决方案。
```python
from collections import deque
# 定义华容道类
class HuaRongDao:
def __init__(self, board):
self.board = board
self.empty_pos = self.get_empty_pos()
# 获取空白位置
def get_empty_pos(self):
for i in range(5):
for j in range(4):
if self.board[i][j] == 0:
return (i, j)
# 移动棋子
def move(self, pos1, pos2):
i1, j1 = pos1
i2, j2 = pos2
self.board[i1][j1], self.board[i2][j2] = self.board[i2][j2], self.board[i1][j1]
# 获取所有可能移动的位置
def get_moves(self):
moves = []
i, j = self.empty_pos
if i > 0:
moves.append((i-1, j))
if i < 4:
moves.append((i+1, j))
if j > 0:
moves.append((i, j-1))
if j < 3:
moves.append((i, j+1))
return moves
# 获取下一步棋盘状态
def get_next_board(self, move):
next_board = [[self.board[i][j] for j in range(4)] for i in range(5)]
self.move(self.empty_pos, move)
next_hrdao = HuaRongDao(next_board)
next_hrdao.empty_pos = move
return next_hrdao
# 判断是否达到目标状态
def is_solved(self):
return self.board[3][1] == 1 and self.board[3][2] == 2
# 广度优先搜索
def bfs(initial_hrdao):
queue = deque([(initial_hrdao, [])])
visited = set()
while queue:
hrdao, moves = queue.popleft()
if hrdao.is_solved():
return moves
visited.add(str(hrdao.board))
for move in hrdao.get_moves():
next_hrdao = hrdao.get_next_board(move)
if str(next_hrdao.board) not in visited:
queue.append((next_hrdao, moves + [move]))
# 棋盘状态
board = [
[1, 1, 0, 2],
[1, 1, 0, 2],
[3, 4, 4, 5],
[6, 7, 8, 5],
[6, 9, 9, 10]
]
# 初始华容道
initial_hrdao = HuaRongDao(board)
# 解题
moves = bfs(initial_hrdao)
print(moves)
```
你可以将程序复制到Python编辑器中运行,得到华容道问题的解决方案。注意,程序中的棋盘状态可以根据实际情况进行修改。
相关推荐
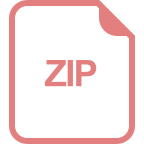
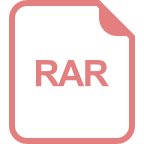
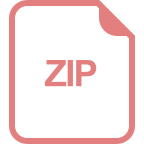














