c++ 读取 二进制文件内的所有数据
时间: 2024-10-15 19:17:10 浏览: 56
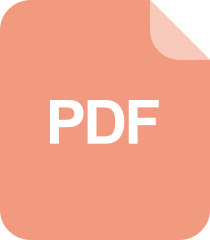
C/C++ 读取16进制文件的方法
在C++中,读取二进制文件内的所有数据通常涉及到使用`fstream`库,特别是`ifstream`(用于输入流)。下面是一个简单的步骤示例:
```cpp
#include <fstream>
#include <vector>
std::vector<char> readBinaryFile(const std::string& filename) {
std::ifstream file(filename, std::ios::binary);
if (!file.is_open()) {
throw std::runtime_error("Failed to open the file");
}
// 计算文件大小
std::streampos fileSize;
file.seekg(0, std::ios::end); // 移动到文件末尾
fileSize = file.tellg(); // 获取当前位置即文件大小
file.seekg(0, std::ios::beg); // 回到开始
// 为存储数据创建一个动态向量
std::vector<char> data(fileSize);
// 读取整个文件内容
file.read(&data[0], fileSize);
// 关闭文件
file.close();
return data;
}
// 使用函数
int main() {
try {
std::vector<char> binaryData = readBinaryFile("your_file.bin");
// 现在binaryData包含了二进制文件的所有数据
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << '\n';
}
return 0;
}
```
在这个例子中,我们首先检查文件是否成功打开,然后获取文件的实际长度,接着创建一个足够大的向量来存放数据。`read()`函数从文件读取指定数量的字节,并存储到向量中。最后别忘了关闭文件。
阅读全文
相关推荐
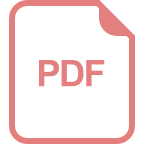
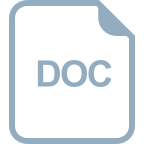
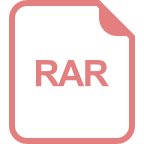
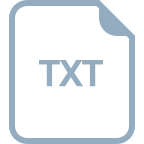
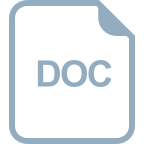
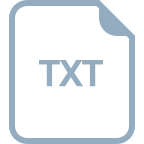
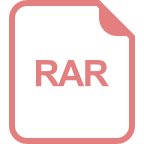
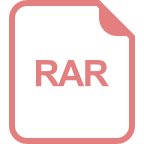
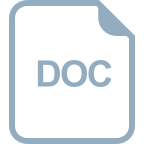
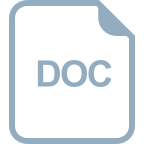


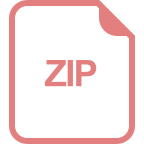
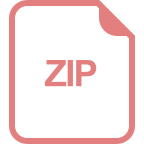