要求编写一个数据结构c++程序来实现判断字符串是否对称,要求同时使用栈和队列来实
时间: 2024-11-15 11:32:02 浏览: 14
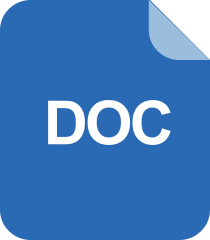
数据结构--栈与队列的应用.doc
为了判断一个字符串是否对称,即正读和反读都一样,我们可以采用双指针法结合栈和队列的数据结构。以下是基本步骤:
1. 使用两个指针,一个从字符串的开始(left),另一个从结束(right)。
2. 创建一个空栈用于存储字符,并将左指针指向的字符入栈。
3. 同时,创建一个队列并放入右指针指向的字符。
4. 当left < right时,循环执行以下操作:
a. 检查栈顶元素是否等于队首元素,如果不等则字符串不是对称的,返回false。
b. 如果相等,则分别出栈和出队,然后将left和right向内移动一位。
5. 当left >= right时,说明所有字符都匹配过,字符串是对称的,返回true。
下面是简单的C++代码实现:
```cpp
#include <iostream>
#include <stack>
#include <queue>
bool isPalindromeWithStackAndQueue(const std::string& str) {
std::stack<char> stack;
std::queue<char> queue;
for (int i = 0; i < str.length(); ++i) {
if (i < str.length() / 2) {
stack.push(str[i]);
queue.push(str[str.length() - i - 1]);
} else if (str[i] == queue.front()) {
queue.pop();
} else {
return false;
}
}
return stack.empty() && queue.empty();
}
int main() {
std::string test_str = "abcba";
if (isPalindromeWithStackAndQueue(test_str)) {
std::cout << "The string is a palindrome.\n";
} else {
std::cout << "The string is not a palindrome.\n";
}
return 0;
}
```
阅读全文
相关推荐
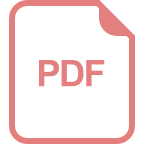
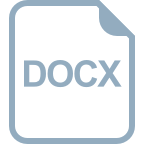

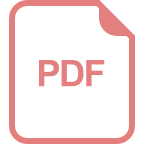
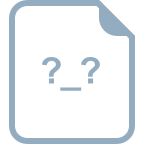
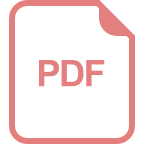
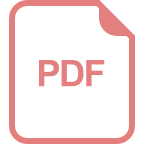
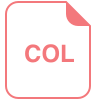
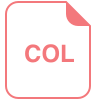
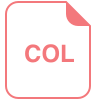
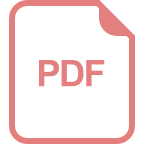
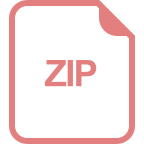
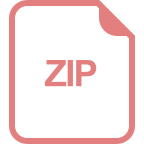
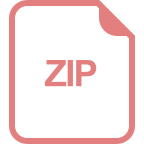
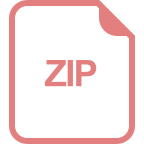
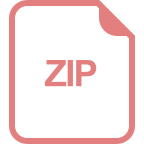