编写一个c++程序来判断字符串是否对称,要求同时使用栈和队列来实现
时间: 2024-11-15 09:32:20 浏览: 16
编写一个C++程序来判断一个字符串是否对称,即正读和反读都一样,可以同时利用栈和队列的数据结构。这里我们先通过队列实现从前往后的遍历,然后利用栈实现从后往前的遍历,比较两个指针所指向的字符是否相等。
```cpp
#include <iostream>
#include <stack>
#include <queue>
#include <string>
bool isPalindromeQueueStack(const std::string& s) {
// 使用队列存储前半部分字符
std::queue<char> front;
for (char c : s) {
front.push(c);
}
// 使用栈存储后半部分字符,并逐个取出与队首比较
std::stack<char> back(s.rbegin(), s.rend());
while (!front.empty() && !back.empty()) {
if (front.front() != back.top()) {
return false;
}
front.pop();
back.pop();
}
return true;
}
int main() {
std::string str = "radar";
if (isPalindromeQueueStack(str)) {
std::cout << "The string is a palindrome.\n";
} else {
std::cout << "The string is not a palindrome.\n";
}
return 0;
}
```
在这个程序中,`isPalindromeQueueStack`函数首先将字符串`s`的前半部分放入队列`front`,然后从后半部分的栈`back`开始取出字符与队列的前端比较。如果发现不匹配,则返回`false`;当两者都遍历完,说明字符串是对称的,返回`true`。
阅读全文
相关推荐
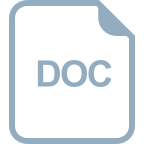
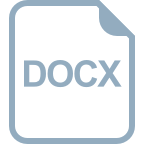
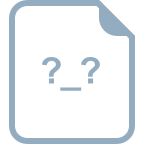

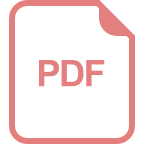
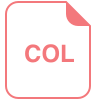
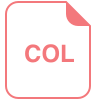
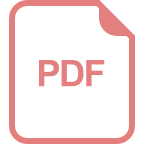
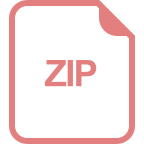
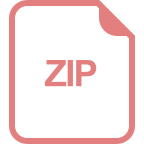
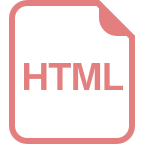
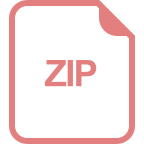
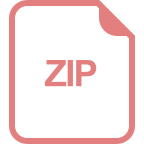
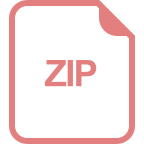
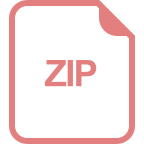
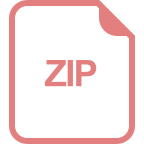
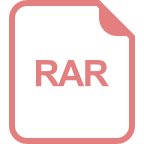