c++计算两个复数的四则运算
时间: 2024-09-11 12:10:35 浏览: 38
在C++中,计算两个复数的四则运算(加法、减法、乘法和除法)需要首先定义复数类,并包含实部(real)和虚部(imaginary)。以下是基本的步骤:
```cpp
#include <iostream>
// 定义复数类
class Complex {
public:
double real; // 实部
double imaginary; // 虚部
// 构造函数
Complex(double r = 0, double i = 0) : real(r), imaginary(i) {}
// 复数加法
Complex operator+(const Complex& other) const {
return Complex(real + other.real, imaginary + other.imaginary);
}
// 减法
Complex operator-(const Complex& other) const {
return Complex(real - other.real, imaginary - other.imaginary);
}
// 乘法
Complex operator*(const Complex& other) const {
return Complex(real * other.real - imaginary * other.imaginary,
real * other.imaginary + imaginary * other.real);
}
// 除法,假设除数非零
Complex operator/(const Complex& other) const {
double denominator = other.real * other.real + other.imaginary * other.imaginary;
return Complex((real * other.real + imaginary * other.imaginary) / denominator,
(imaginary * other.real - real * other.imaginary) / denominator);
}
};
int main() {
Complex num1(3, 4); // 复数3 + 4i
Complex num2(1, -2); // 复数1 - 2i
// 执行四则运算并打印结果
Complex sum = num1 + num2;
Complex difference = num1 - num2;
Complex product = num1 * num2;
if (product.real != 0 && product.imaginary != 0) { // 防止除数为零
Complex quotient = num1 / num2;
std::cout << "Sum: " << sum.real << "+" << sum.imaginary << "i\n";
std::cout << "Difference: " << difference.real << "+" << difference.imaginary << "i\n";
std::cout << "Product: " << product.real << "+" << product.imaginary << "i\n";
std::cout << "Quotient: " << quotient.real << "+" << quotient.imaginary << "i\n";
} else {
std::cout << "Cannot divide by zero.\n";
}
return 0;
}
```
阅读全文
相关推荐
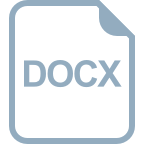
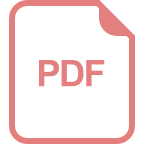
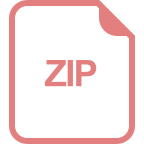
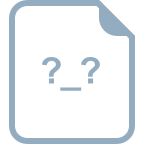
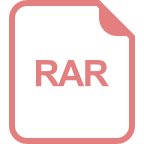
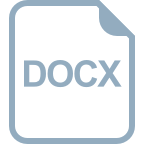
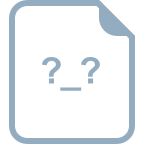
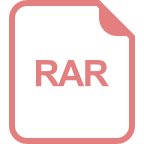
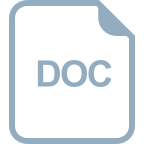
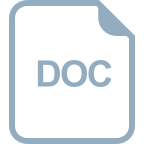






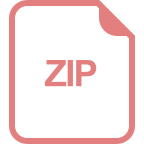