数据结构c语言用串实现kmp
时间: 2023-11-25 15:08:19 浏览: 83
以下是使用C语言实现KMP算法的代码,其中SString是一个自定义的串类型:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAXSIZE 100
typedef struct {
char ch[MAXSIZE];
int length;
} SString;
// 获取next数组
void GetNext(SString T, int *next) {
int i = 1, j = 0;
next[1] = 0;
while (i < T.length) {
if (j == 0 || T.ch[i - 1] == T.ch[j - 1]) {
i++;
j++;
next[i] = j;
} else {
j = next[j];
}
}
}
// KMP算法
int Index_KMP(SString S, SString T, int pos) {
int i = pos, j = 1;
int next[T.length + 1];
GetNext(T, next);
while (i <= S.length && j <= T.length) {
if (j == 0 || S.ch[i - 1] == T.ch[j - 1]) {
i++;
j++;
} else {
j = next[j];
}
}
if (j > T.length) {
return i - T.length;
} else {
return 0;
}
}
int main() {
SString S = {"ABCABCE", 7};
SString T = {"ABCE", 4};
int pos = Index_KMP(S, T, 1);
if (pos) {
printf("匹配成功,位置为:%d\n", pos);
} else {
printf("匹配失败\n");
}
return 0;
}
```
阅读全文
相关推荐








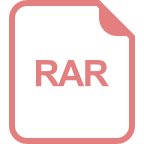







