C#使用WMI启用远程主机上的服务
时间: 2024-10-23 22:09:12 浏览: 21
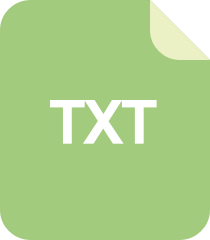
c#利用wmi远程关机

在C#中,Windows Management Instrumentation (WMI) 提供了一种强大的机制来管理和控制远程计算机的服务。要通过WMI启用远程主机上的服务,你需要使用命名空间`root\cimv2\win32`下的`Win32_Service`类,并确保你的应用程序有适当的权限来进行远程操作。
以下是一个简单的步骤示例:
```csharp
using System;
using System.Management;
namespace RemoteServiceControl
{
class Program
{
static void Main(string[] args)
{
// 创建连接字符串,包括计算机名和安全凭据(如果需要)
string connectionString = "Winmgmts:\\\\{remoteComputerName}\\root\\cimv2";
ConnectionOptions options = new ConnectionOptions();
if (!string.IsNullOrEmpty(username) && !string.IsNullOrEmpty(password))
{
options.Username = username;
options.Password = password;
options.ImpersonationLevel = ImpersonationLevel.Impersonate; // 如果需要以其他用户身份连接
}
// 使用连接选项建立管理对象
ManagementScope scope = new ManagementScope(connectionString, options);
try
{
// 定义要操作的服务名称
string serviceName = "YourServiceName";
// 创建Service对象实例
ManagementObject serviceObj = new ManagementObject(scope, $"SELECT * FROM Win32_Service WHERE Name = '{serviceName}'", null);
// 检查服务是否已启动
bool isRunning = Convert.ToBoolean(serviceObj.InvokeMethod("Start"));
if (isRunning)
Console.WriteLine($"Service {serviceName} on remote host started successfully.");
else
Console.WriteLine($"Failed to start service {serviceName} on the remote host.");
// 关闭连接
serviceObj.Close();
}
catch (Exception ex)
{
Console.WriteLine($"Error occurred: {ex.Message}");
}
}
}
}
```
记得替换`remoteComputerName`、`username`和`password`(如果有)为实际值。执行此代码前,请确保你的应用程序具有足够的权限访问远程计算机上的服务。
阅读全文
相关推荐
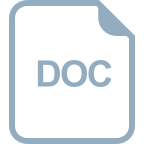
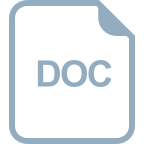




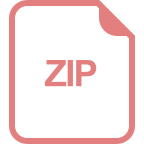
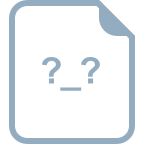
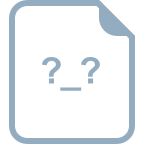
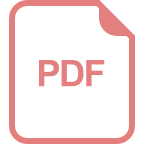
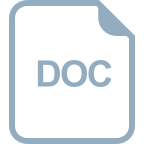
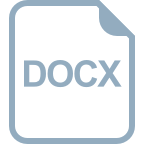
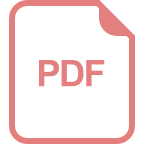
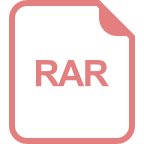
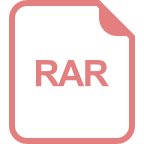
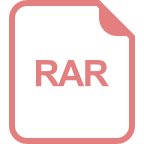
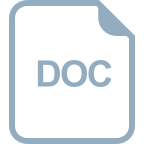
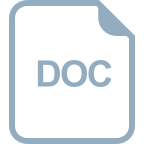