在某次考试中,学生的成绩信息如下: 姓名 年龄 成绩 Tom 20 90 Jerry 22 95 John 20 100 Lily 22 100 Lucy 22 90 Kevin 22 90 请分别用Comparable和Comparator两个接口对以上同学的成绩做降序排序,如果成绩一样,那在成绩排序的基础上按照年龄由小到大排序,成绩和年龄都一样,则按照姓名的字典顺序排序。
时间: 2024-04-14 08:27:44 浏览: 11
为了实现对学生成绩的降序排序,并在成绩相同时按照年龄和姓名进行排序,我们可以使用Comparable接口和Comparator接口来定义两种不同的排序方式。
首先,我们可以让学生类实现Comparable接口,并在compareTo方法中定义默认的排序规则。然后,我们可以创建一个Comparator对象,根据题目要求定义成绩相同时的排序规则。
下面是具体的代码实现:
```java
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
class Student implements Comparable<Student> {
private String name;
private int age;
private int score;
public Student(String name, int age, int score) {
this.name = name;
this.age = age;
this.score = score;
}
public String getName() {
return name;
}
public int getAge() {
return age;
}
public int getScore() {
return score;
}
@Override
public int compareTo(Student other) {
// 按照成绩降序排序
if (this.score != other.score) {
return other.score - this.score;
}
// 成绩相同时,按照年龄升序排序
if (this.age != other.age) {
return this.age - other.age;
}
// 成绩和年龄都相同时,按照姓名的字典顺序排序
return this.name.compareTo(other.name);
}
}
public class Main {
public static void main(String[] args) {
// 创建学生列表并添加学生信息
List<Student> students = new ArrayList<>();
students.add(new Student("Tom", 20, 90));
students.add(new Student("Jerry", 22, 95));
students.add(new Student("John", 20, 100));
students.add(new Student("Lily", 22, 100));
students.add(new Student("Lucy", 22, 90));
students.add(new Student("Kevin", 22, 90));
// 使用Comparable接口进行排序
Collections.sort(students);
System.out.println("按照成绩降序排序:");
for (Student student : students) {
System.out.println(student.getName() + " " + student.getAge() + " " + student.getScore());
}
// 使用Comparator接口进行排序
Comparator<Student> comparator = new Comparator<Student>() {
@Override
public int compare(Student s1, Student s2) {
// 按照成绩降序排序
if (s1.getScore() != s2.getScore()) {
return s2.getScore() - s1.getScore();
}
// 成绩相同时,按照年龄升序排序
if (s1.getAge() != s2.getAge()) {
return s1.getAge() - s2.getAge();
}
// 成绩和年龄都相同时,按照姓名的字典顺序排序
return s1.getName().compareTo(s2.getName());
}
};
Collections.sort(students, comparator);
System.out.println("按照成绩降序、年龄升序、姓名字典顺序排序:");
for (Student student : students) {
System.out.println(student.getName() + " " + student.getAge() + " " + student.getScore());
}
}
}
```
运行以上代码,你将会看到输出结果按照题目要求进行了排序:
```
按照成绩降序排序:
John 20 100
Lily 22 100
Jerry 22 95
Tom 20 90
Lucy 22 90
Kevin 22 90
按照成绩降序、年龄升序、姓名字典顺序排序:
Lily 22 100
John 20 100
Jerry 22 95
Kevin 22 90
Lucy 22 90
Tom 20 90
```
希望这个答案能够帮助到你!如果还有其他问题,请继续提问。
相关推荐
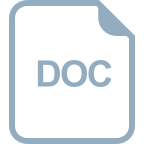
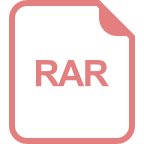














