采用头插法建立单链表,当输入0时,创建结束,并实现遍历链表和对其翻转的功能,c语言
时间: 2024-10-19 13:15:52 浏览: 39
在C语言中,我们可以使用头插法构建单链表并实现遍历、插入节点以及反转链表的操作。这里是一个简单的示例:
首先,我们定义一个结构体`Node`表示链表节点,包含数据域和指向下一个节点的指针:
```c
typedef struct Node {
int data;
struct Node* next;
} Node;
```
接下来,我们创建链表的方法,包括初始化链表和头插法插入节点:
```c
// 初始化空链表
Node* createList() {
return (Node*)malloc(sizeof(Node));
}
// 插入节点到链表头部
void insertAtHead(Node** head, int data) {
Node* newNode = createList();
newNode->data = data;
newNode->next = *head;
*head = newNode;
}
```
对于遍历链表,可以使用一个辅助函数`displayList`:
```c
void displayList(Node* head) {
Node* current = head;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
```
要反转链表,我们需要两个指针,一个指向当前节点,另一个指向前一个节点:
```c
Node* reverseList(Node* head) {
Node* prev = NULL;
Node* current = head;
Node* next;
while (current != NULL) {
next = current->next; // 保存当前节点的下一个节点
current->next = prev; // 将当前节点的next指向前一个节点
prev = current; // 移动prev指针
current = next; // 移动current指针
}
return prev; // 返回新的头节点
}
```
结合以上代码,你可以通过以下方式创建链表、插入节点并进行操作:
```c
int main() {
Node* head = createList(); // 创建空链表
// 插入节点示例
insertAtHead(&head, 5); // 链表: 5 -> NULL
insertAtHead(&head, 4); // 链表: 4 -> 5 -> NULL
insertAtHead(&head, 3); // 链表: 3 -> 4 -> 5 -> NULL
// 遍历链表
displayList(head); // 输出: 3 4 5
// 反转链表
head = reverseList(head); // 翻转后的链表: 5 -> 4 -> 3
// 再次遍历显示反转后的链表
displayList(head); // 输出: 5 4 3
return 0;
}
```
阅读全文
相关推荐
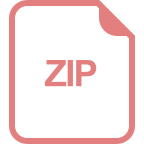
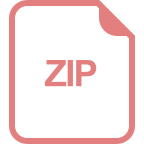
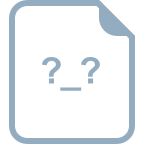
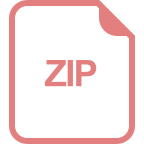
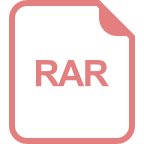
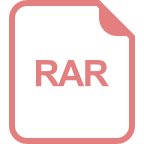
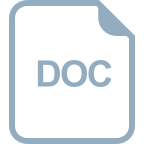
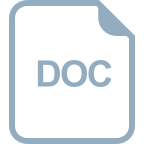
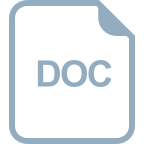
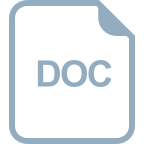
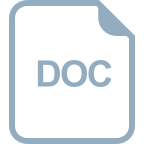
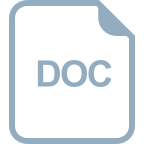
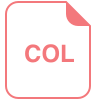





