试编程实现基于基尼指数进行划分选择的决策树算法
时间: 2024-12-06 13:33:38 浏览: 22
基尼指数是一种用于衡量数据纯度的指标,在构建决策树中常用于节点分裂的选择。为了实现一个简单的基于基尼指数的决策树算法,我们可以采用递归的方式。这里是一个基本的Python示例:
```python
import numpy as np
class Node:
def __init__(self, feature=None, threshold=None, left=None, right=None):
self.feature = feature
self.threshold = threshold
self.left = left
self.right = right
def gini_index(data):
classes, counts = np.unique(data.target, return_counts=True)
impurity = sum((count / len(data)) * (1 - count / len(data)) for count in counts)
return impurity
def entropy_index(data):
# 这里是信息熵的计算方式,如果只考虑基尼指数,则不需要这个函数
pass
def find_best_split(data, features, labels):
best_gain = 0
best_feature, best_threshold = None, None
for feature in features:
thresholds = data[feature].unique()
for threshold in thresholds:
left_data, right_data = split_dataset(data, feature, threshold)
if len(left_data) == 0 or len(right_data) == 0:
continue
gain = gini_index(data) - gini_index(left_data) * len(left_data) / len(data) - gini_index(right_data) * len(right_data) / len(data)
if gain > best_gain:
best_gain = gain
best_feature, best_threshold = feature, threshold
return best_feature, best_threshold
def split_dataset(data, feature, threshold):
return data[data[feature] <= threshold], data[data[feature] > threshold]
def build_tree(data, depth=0, max_depth=None):
features = list(data.columns[:-1]) # 假设最后一列是目标变量
if max_depth and depth >= max_depth:
return Node(feature=np.argmax(np.bincount(data.iloc[:, -1])))
best_feature, best_threshold = find_best_split(data, features, data.iloc[:, -1])
node = Node(feature=best_feature, threshold=best_threshold)
feature_data = data[[best_feature]]
left_data, right_data = split_dataset(data, best_feature, best_threshold)
node.left = build_tree(left_data, depth + 1, max_depth)
node.right = build_tree(right_data, depth + 1, max_depth)
return node
# 使用示例
data = ... # 包含特征和标签的数据集
max_depth = 5 # 决策树的最大深度
tree = build_tree(data, max_depth=max_depth)
```
这个代码首先定义了节点类,然后实现了计算基尼指数、寻找最优分割点以及递归构建决策树的过程。注意这只是一个简化版本,实际应用中可能需要处理更多细节,比如处理缺失值、类别不平衡等。
阅读全文
相关推荐
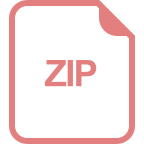




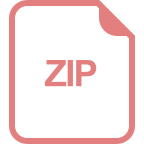
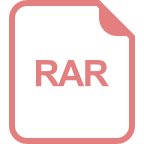
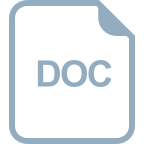
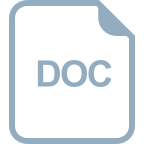
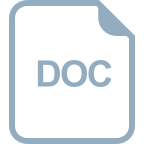
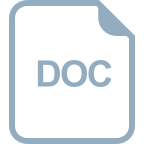
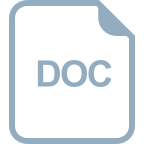
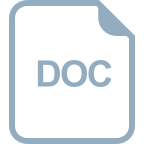
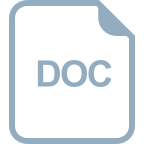
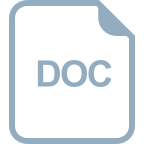
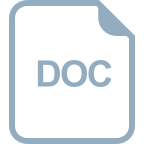
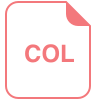
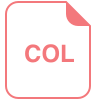