python凯撒密码解密
时间: 2023-10-17 18:32:20 浏览: 279
你可以使用以下代码来解密凯撒密码:
```python
def caesar_decrypt(ciphertext, shift):
plaintext = ""
for char in ciphertext:
if char.isalpha():
ascii_offset = ord('A') if char.isupper() else ord('a')
decrypted_char = chr((ord(char) - ascii_offset - shift) % 26 + ascii_offset)
plaintext += decrypted_char
else:
plaintext += char
return plaintext
ciphertext = "Xli riizivw xlmw xs irgvctxih xlex mqi ryqfiv"
shift = 4
decrypted_text = caesar_decrypt(ciphertext, shift)
print(decrypted_text)
```
在这个例子中,我们假设密文是大写字母和空格组成的,解密后的明文将保持相同的字母大小写和空格。
解密结果应该是:"The password must be entered without any spaces"。
相关问题
python 凯撒密码解密
Python中的凯撒密码解密可以通过以下步骤实现:
1. 获取密文和偏移量:首先,你需要获取凯撒密码的密文和偏移量。密文是被加密的文本,而偏移量是用于解密的数字,表示字母向后移动的位数。
2. 解密算法:凯撒密码的解密算法很简单,只需要将每个字母向前移动偏移量个位置即可。但需要注意的是,如果向前移动超过了字母表的边界,需要回到字母表的开头继续计算。
3. 实现代码:下面是一个简单的Python代码示例,用于解密凯撒密码:
```python
def caesar_decrypt(ciphertext, offset):
plaintext = ""
for char in ciphertext:
if char.isalpha():
ascii_offset = ord('a') if char.islower() else ord('A')
decrypted_char = chr((ord(char) - ascii_offset - offset) % 26 + ascii_offset)
plaintext += decrypted_char
else:
plaintext += char
return plaintext
# 示例使用
ciphertext = "L zwjrw jvsqflk qeb ixwixl"
offset = 5
plaintext = caesar_decrypt(ciphertext, offset)
print("解密结果:", plaintext)
```
这段代码中,`caesar_decrypt`函数接受两个参数:`ciphertext`表示密文,`offset`表示偏移量。函数通过遍历密文中的每个字符,判断是否为字母,然后根据偏移量进行解密操作。最后返回解密后的明文。
用Python凯撒密码解密
可以使用以下代码解密凯撒密码:
```python
def caesar_decrypt(ciphertext, shift):
plaintext = ""
for char in ciphertext:
if char.isalpha():
char_code = ord(char)
char_code -= shift
if char.isupper():
if char_code < ord('A'):
char_code += 26
elif char_code > ord('Z'):
char_code -= 26
else:
if char_code < ord('a'):
char_code += 26
elif char_code > ord('z'):
char_code -= 26
plaintext += chr(char_code)
else:
plaintext += char
return plaintext
```
其中,ciphertext 是密文,shift 是密钥,表示字母向后移动的位数。例如,如果 shift 为 3,则 A 变成 D,B 变成 E,以此类推。
阅读全文
相关推荐
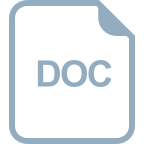
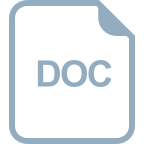
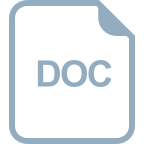









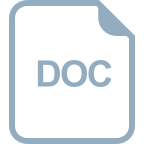
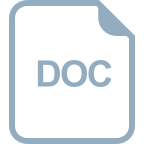
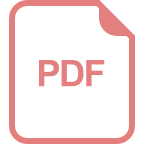
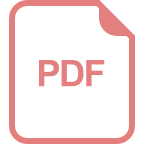