python用reshape降一维
时间: 2023-07-17 11:12:23 浏览: 93
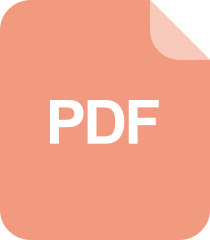
python矩阵转换为一维数组的实例
使用numpy库中的reshape函数可以将数组降维。以下是一个示例代码:
```python
import numpy as np
# 创建一个二维数组
arr = np.array([[1, 2, 3], [4, 5, 6]])
print("原始数组:")
print(arr)
# 使用reshape函数将数组降为一维
arr_1d = np.reshape(arr, -1)
print("降为一维后的数组:")
print(arr_1d)
```
输出结果:
```
原始数组:
[[1 2 3]
[4 5 6]]
降为一维后的数组:
[1 2 3 4 5 6]
```
在reshape函数中,可以传入参数-1表示自动计算该轴的长度,这样可以方便地将多维数组降为一维。
阅读全文
相关推荐
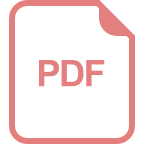
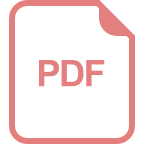















