量子粒子群 python代码
时间: 2023-11-08 20:04:51 浏览: 51
以下是一个简单的量子粒子群算法的 Python 代码示例:
```python
import numpy as np
# 目标函数
def fitness_func(x):
return np.sum(x**2)
# 初始化粒子群
def init_swarm(n_particles, n_dimensions):
swarm = np.random.rand(n_particles, n_dimensions)
return swarm
# 更新速度和位置
def update(swarm, velocity, pbest, gbest, w, c1, c2):
r1 = np.random.rand(*swarm.shape)
r2 = np.random.rand(*swarm.shape)
velocity = w * velocity + c1 * r1 * (pbest - swarm) + c2 * r2 * (gbest - swarm)
swarm = swarm + velocity
return swarm, velocity
# 量子粒子群算法
def qpswarm(n_particles, n_dimensions, max_iter):
# 初始化粒子群
swarm = init_swarm(n_particles, n_dimensions)
velocity = np.zeros_like(swarm)
pbest = swarm.copy()
pbest_fitness = np.zeros(n_particles)
gbest = np.zeros(n_dimensions)
gbest_fitness = np.inf
# 迭代
for i in range(max_iter):
# 计算适应度
fitness = np.apply_along_axis(fitness_func, 1, swarm)
# 更新个体最优解
mask = fitness < pbest_fitness
pbest[mask] = swarm[mask]
pbest_fitness[mask] = fitness[mask]
# 更新全局最优解
idx = np.argmin(pbest_fitness)
if pbest_fitness[idx] < gbest_fitness:
gbest = pbest[idx]
gbest_fitness = pbest_fitness[idx]
# 更新速度和位置
swarm, velocity = update(swarm, velocity, pbest, gbest, 0.5, 1.0, 1.0)
return gbest, gbest_fitness
# 示例
gbest, gbest_fitness = qpswarm(50, 10, 100)
print("Global best solution:", gbest)
print("Global best fitness:", gbest_fitness)
```
相关推荐
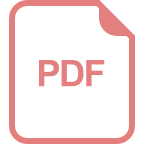














