使用python实现线性回归
时间: 2024-01-01 07:07:01 浏览: 33
要使用Python实现线性回归,你可以使用scikit-learn库。下面是一个简单的示例代码:
```python
import numpy as np
from sklearn.linear_model import LinearRegression
# 创建一些样本数据
X = np.array([[1, 1], [1, 2], [2, 2], [2, 3]])
y = np.dot(X, np.array([1, 2])) + 3
# 创建线性回归模型并进行训练
model = LinearRegression()
model.fit(X, y)
# 输出模型的系数和截距
print("Coefficients: ", model.coef_)
print("Intercept: ", model.intercept_)
# 进行预测
X_test = np.array([[3, 4]])
y_pred = model.predict(X_test)
print("Predicted y: ", y_pred)
```
这段代码创建了一个简单的线性回归模型,通过使用`LinearRegression`类来构建模型。然后使用`fit`方法传入特征矩阵`X`和目标向量`y`来进行训练。最后,可以使用`predict`方法对新的样本进行预测。
希望对你有所帮助!如果有任何问题,请随时问我。
相关问题
使用python实现线性回归算法
以下是使用Python实现线性回归算法的步骤和代码:
1.导入必要的库和数据集
```python
import numpy as np
from sklearn.datasets import make_regression
from sklearn.model_selection import train_test_split
```
2.生成数据集
```python
X, y = make_regression(n_samples=500, n_features=1, noise=20, random_state=4)
```
3.将数据集分为训练集和测试集
```python
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0)
```
4.定义线性回归模型
```python
class LinearRegression:
def __init__(self, lr=0.01, n_iters=1000):
self.lr = lr
self.n_iters = n_iters
self.weights = None
self.bias = None
def fit(self, X, y):
# 初始化参数
n_samples, n_features = X.shape
self.weights = np.zeros(n_features)
self.bias = 0
# 梯度下降
for _ in range(self.n_iters):
y_predicted = np.dot(X, self.weights) + self.bias
dw = (1 / n_samples) * np.dot(X.T, (y_predicted - y))
db = (1 / n_samples) * np.sum(y_predicted - y)
self.weights -= self.lr * dw
self.bias -= self.lr * db
def predict(self, X):
y_predicted = np.dot(X, self.weights) + self.bias
return y_predicted
```
5.训练模型并预测结果
```python
regressor = LinearRegression(lr=0.01, n_iters=1000)
regressor.fit(X_train, y_train)
predictions = regressor.predict(X_test)
```
6.评估模型
```python
def mse(y_true, y_pred):
return np.mean((y_true - y_pred) ** 2)
print("Mean Squared Error:", mse(y_test, predictions))
```
用python实现线性回归
下面是一个简单的例子,使用Python实现线性回归:
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义输入数据
X = np.array([1, 2, 3, 4, 5])
Y = np.array([4, 7, 10, 13, 16])
# 计算输入数据的平均值
mean_x = np.mean(X)
mean_y = np.mean(Y)
# 计算斜率和截距
numerator = np.sum((X - mean_x) * (Y - mean_y))
denominator = np.sum((X - mean_x) ** 2)
m = numerator / denominator
b = mean_y - m * mean_x
# 绘制拟合直线
plt.scatter(X, Y)
plt.plot(X, m * X + b, color='red')
plt.show()
```
在上面的代码中,我们首先定义了输入数据X和Y。接下来,我们计算X和Y的平均值,并使用这些值计算斜率和截距。最后,我们使用matplotlib库绘制拟合直线。
相关推荐
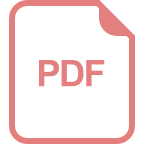












