用java的类与对象编写复数的加减乘除
时间: 2024-10-25 16:09:26 浏览: 14
在Java中,我们可以创建一个表示复数的类`ComplexNumber`,包含两个属性:实部(`real`)和虚部(`imaginary`),然后提供加、减、乘、除的方法来操作复数。下面是一个简单的示例:
```java
public class ComplexNumber {
private double real;
private double imaginary;
// 构造函数
public ComplexNumber(double real, double imaginary) {
this.real = real;
this.imaginary = imaginary;
}
// Getters and Setters
public double getReal() {
return real;
}
public void setReal(double real) {
this.real = real;
}
public double getImaginary() {
return imaginary;
}
public void setImaginary(double imaginary) {
this.imaginary = imaginary;
}
// 复数加法
public ComplexNumber add(ComplexNumber other) {
double newReal = this.real + other.getReal();
double newImaginary = this.imaginary + other.getImaginary();
return new ComplexNumber(newReal, newImaginary);
}
// 复数减法
public ComplexNumber subtract(ComplexNumber other) {
double newReal = this.real - other.getReal();
double newImaginary = this.imaginary - other.getImaginary();
return new ComplexNumber(newReal, newImaginary);
}
// 复数乘法
public ComplexNumber multiply(ComplexNumber other) {
double realProduct = this.real * other.getReal() - this.imaginary * other.getImaginary();
double imagProduct = this.real * other.getImaginary() + this.imaginary * other.getReal();
return new ComplexNumber(realProduct, imagProduct);
}
// 复数除法 (假设除数不为0)
public ComplexNumber divide(ComplexNumber divisor) {
double denominator = divisor.getReal() * divisor.getReal() + divisor.getImaginary() * divisor.getImaginary();
double newReal = (this.real * divisor.getReal() + this.imaginary * divisor.getImaginary()) / denominator;
double newImaginary = (this.imaginary * divisor.getReal() - this.real * divisor.getImaginary()) / denominator;
return new ComplexNumber(newReal, newImaginary);
}
}
```
阅读全文
相关推荐
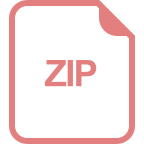
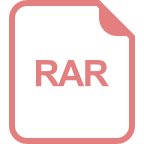






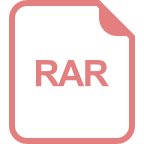
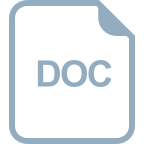
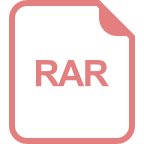
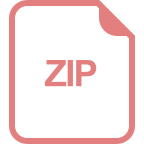
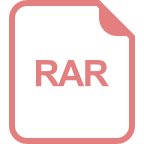
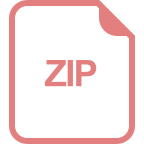
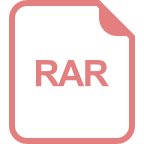
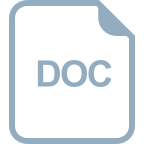

