f snake_matrix(n): matrix = [[0] * n for _ in range(n)] # 创建一个空的 n x n 矩阵 num = 1 # 从1开始填充矩阵 for i in range(n): if i % 2 == 0: for j in range(i+1): matrix[i-j][j] = num num += 1 else: for j in range(i+1): matrix[j][i-j] = num num += 1 return matrixdef print_snake_matrix(matrix): for row in matrix: for num in row: if num != 0: print(num, end=' ') print()n = int(input("请输入一个正整数N:"))matrix = snake_matrix(n)print_snake_matrix(matrix)
时间: 2024-02-14 15:30:48 浏览: 26
这段代码定义了一个名为`snake_matrix`的函数,它接受一个参数 `n`。函数内部首先创建了一个空的 n x n 矩阵,并且从1开始填充矩阵。填充方式是按照蛇形的顺序进行填充,从左上角开始逐行填充,当行数是奇数时,填充方向是从右下到左上,当行数是偶数时,填充方向是从左上到右下。最后,函数返回填充完成的矩阵。
接下来定义了一个名为`print_snake_matrix`的函数,它接受一个参数 `matrix`,用于打印矩阵。函数内部使用嵌套循环遍历矩阵的每个元素,并打印非零元素。最后,根据用户输入的正整数 N,调用`snake_matrix`函数创建矩阵并调用`print_snake_matrix`函数打印矩阵。
请注意,这段代码中的输入操作需要在 Python 环境中运行。如果你有任何问题,请随时提问!
相关问题
def snake_matrix(n): matrix = [[0] * n for _ in range(n)] # 创建一个空的 n x n 矩阵 num = 1 # 从1开始填充矩阵 for i in range(n): if i % 2 == 0: for j in range(i+1): matrix[i-j][j] = num num += 1 else: for j in range(i+1): matrix[j][i-j] = num num += 1 return matrix
这是一个函数,名为`snake_matrix`,它接受一个参数`n`,用于确定矩阵的大小为 n x n。函数首先创建一个空的 n x n 矩阵,然后从1开始填充这个矩阵。
接下来,函数使用一个循环来遍历矩阵的每一行。如果当前行的索引是偶数,就从左上角开始向右下角填充数字;如果当前行的索引是奇数,就从左下角开始向右上角填充数字。每填充一个数字,就将`num`增加1。最后,函数返回填充完毕的矩阵。
这个函数实现了一个蛇形矩阵的生成算法,它将数字按照蛇形的方式填充到矩阵中。
Give a positive integer n not greater than 9 and output a “Snake matrix” with n rows and n columns. For example: Input: 4 output:
Sure, I can help you with that! Here's a Python code that generates a Snake matrix with n rows and n columns for a positive integer n not greater than 9:
```
n = int(input("Enter a positive integer not greater than 9: "))
matrix = [[0] * n for i in range(n)]
num = 1
for i in range(n):
if i % 2 == 0:
for j in range(n):
matrix[i][j] = num
num += 1
else:
for j in range(n-1, -1, -1):
matrix[i][j] = num
num += 1
for row in matrix:
print(row)
```
This code first prompts the user to enter a positive integer not greater than 9, creates an empty matrix of size n x n, and then fills in the matrix in a "snake" pattern by iterating over each row and column. Finally, it prints the matrix to the console.
For example, if the user enters 4, the output will be:
```
[1, 2, 3, 4]
[8, 7, 6, 5]
[9, 10, 11, 12]
[16, 15, 14, 13]
```
I hope this helps! Let me know if you have any other questions.
相关推荐
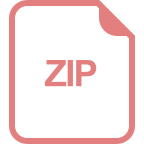











