帮我写一个迷宫路径 广度优先算法C++
时间: 2024-12-24 21:22:38 浏览: 1
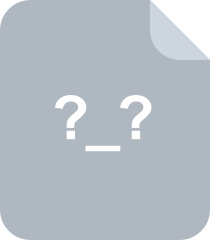
基于C++语言的广度优先算法的迷宫问题程序源码

当然可以,广度优先搜索(Breadth-First Search,BFS)是一种用于遍历或寻找图中所有节点的经典算法,特别是在解决迷宫问题时非常有效。以下是一个简单的C++实现,用于找出从起点到终点的最短路径:
```cpp
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
// 定义迷宫矩阵
vector<vector<char>> maze = {
{'0', '1', '0', '0'},
{'0', '0', '0', '1'},
{'0', '0', '0', '0'},
{'0', '1', '0', '0'}
};
// 细化网格大小(这里假设每个单元格是1x1)
const int ROWS = maze.size();
const int COLS = maze[0].size();
// 检查是否越界,并标记已访问的节点
bool isValid(int i, int j) {
return (i >= 0 && i < ROWS && j >= 0 && j < COLS && maze[i][j] == '0');
}
// 遍历并打印路径
void printPath(vector<pair<int, int>>& path) {
if (path.empty()) {
cout << "No path found.\n";
} else {
for (int i = path.size() - 1; i >= 0; --i) {
int x = path[i].first;
int y = path[i].second;
cout << "(" << x << ", " << y << ") ";
}
cout << "-> Start\n";
}
}
// 广度优先搜索函数
void bfs(int startRow, int startCol, vector<pair<int, int>>& path) {
// 初始化队列和已访问数组
queue<pair<int, int>> q;
vector<bool> visited(ROWS * COLS, false);
q.push({startRow, startCol});
visited[startRow * COLS + startCol] = true;
while (!q.empty()) {
pair<int, int> current = q.front();
q.pop();
if (current == make_pair(ROWS - 1, COLS - 1)) { // 到达终点
printPath(path);
return;
} else {
// 检查相邻位置
int neighbors[] = {current.first + 1, current.first - 1, current.second + 1, current.second - 1};
for (int neighbor : neighbors) {
int row = current.first, col = current.second;
if (isValid(row, col) && !visited[row * COLS + col]) {
visited[row * COLS + col] = true;
path.push_back(current);
q.push({row, col});
}
}
}
}
}
int main() {
int startRow = 0, startCol = 0; // 例如起点是左上角
vector<pair<int, int>> path;
bfs(startRow, startCol, path);
return 0;
}
```
在这个例子中,我们首先定义了一个迷宫矩阵`maze`,然后通过`bfs`函数从起点开始进行探索。当找到终点时,它会记录下到达每一步的位置,并返回结果。记得将`startRow`和`startCol`替换为你实际的迷宫起点。
阅读全文
相关推荐
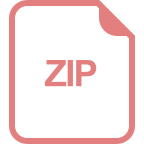
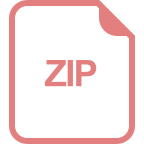




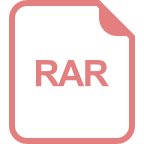
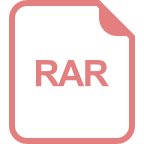
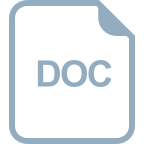
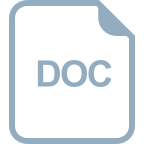
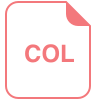





