走迷宫问题c++广度优先算法
时间: 2023-08-11 22:24:42 浏览: 56
使用广度优先搜索来解决迷宫问题需要借助一个队列来实现。以下是一个基于队列的 C++ 实现示例:
```c++
#include <iostream>
#include <vector>
#include <queue>
using namespace std;
// 定义迷宫类型
typedef vector<vector<int>> Maze;
// 定义状态类型
struct State {
int x, y, step;
};
// 定义方向数组
const int dx[] = {0, 0, 1, -1};
const int dy[] = {1, -1, 0, 0};
// 判断是否越界
bool isValid(const Maze& maze, int x, int y) {
return x >= 0 && x < maze.size() && y >= 0 && y < maze[0].size() && maze[x][y] == 0;
}
// 广度优先搜索
int bfs(Maze& maze, int sx, int sy, int ex, int ey) {
queue<State> q;
q.push({sx, sy, 0}); // 将起点入队
maze[sx][sy] = -1; // 标记为已访问
while (!q.empty()) {
State s = q.front(); q.pop();
if (s.x == ex && s.y == ey) { // 到达终点
return s.step;
}
for (int i = 0; i < 4; i++) { // 尝试四个方向
int nx = s.x + dx[i], ny = s.y + dy[i];
if (isValid(maze, nx, ny)) {
q.push({nx, ny, s.step + 1}); // 将新状态入队
maze[nx][ny] = -1; // 标记为已访问
}
}
}
return -1; // 无解
}
int main() {
// 读入迷宫
int n, m;
cin >> n >> m;
Maze maze(n, vector<int>(m));
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
cin >> maze[i][j];
}
}
// 搜索
int steps = bfs(maze, 0, 0, n - 1, m - 1);
if (steps >= 0) {
cout << steps << endl;
} else {
cout << "No" << endl;
}
return 0;
}
```
在上面的实现中,我们使用一个结构体来表示状态,包括当前位置坐标和已经走的步数。在搜索过程中,我们使用一个队列来保存状态,从起点开始,依次尝试四个方向,将新状态加入队列中,直到到达终点或者队列为空为止。搜索过程中,我们使用 -1 表示已访问状态,0 表示未访问状态。如果找到了到终点的路径,则返回路径长度,否则返回 -1 表示无解。
相关推荐
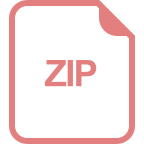
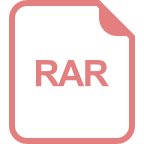
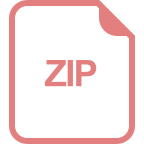
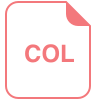
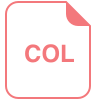
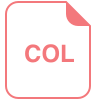
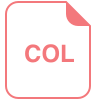
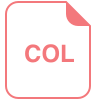









