c++走迷宫游戏代码
时间: 2023-12-10 18:33:42 浏览: 39
以下是一个简单的C++迷宫游戏代码,使用了深度优先搜索算法来寻找迷宫出口:
```c++
#include <iostream>
#include <stack>
using namespace std;
const int N = 10; // 迷宫大小
int maze[N][N] = { // 迷宫地图
{1,1,1,1,1,1,1,1,1,1},
{1,0,0,1,0,0,0,1,0,1},
{1,0,0,1,0,0,0,1,0,1},
{1,0,0,0,0,1,1,0,0,1},
{1,0,1,1,1,0,0,0,0,1},
{1,0,0,0,1,0,0,0,0,1},
{1,0,1,0,0,0,1,0,0,1},
{1,0,1,1,1,0,1,1,0,1},
{1,1,0,0,0,0,0,0,0,1},
{1,1,1,1,1,1,1,1,1,1}
};
struct Point { // 表示一个点的结构体
int x, y;
Point(int _x, int _y) : x(_x), y(_y) {}
};
stack<Point> path; // 用于存储路径的栈
bool dfs(int x, int y) { // 深度优先搜索
if (maze[x][y] == 0) { // 如果当前位置是通路
maze[x][y] = 2; // 标记为已经走过
path.push(Point(x, y)); // 将当前位置加入路径栈中
if (x == N-2 && y == N-2) { // 如果到达终点
return true;
}
if (dfs(x+1, y) || dfs(x, y+1) || dfs(x-1, y) || dfs(x, y-1)) { // 按照右、下、左、上的顺序搜索
return true;
}
path.pop(); // 如果四个方向都走不通,将当前位置从路径栈中弹出
}
return false;
}
int main() {
if (dfs(1, 1)) { // 从起点开始搜索
cout << "找到了一条路径:" << endl;
while (!path.empty()) { // 输出路径
Point p = path.top();
path.pop();
cout << "(" << p.x << ", " << p.y << ")" << endl;
}
} else {
cout << "没有找到路径!" << endl;
}
return 0;
}
```
相关推荐
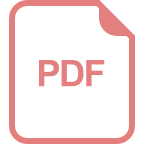
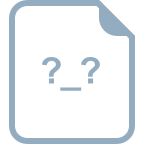
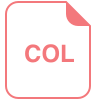
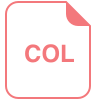











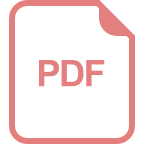