用c语言写一个顺序表的插入与删除
时间: 2024-09-24 07:01:43 浏览: 34
在C语言中,我们可以使用结构体来创建一个简单的顺序表(数组实现)。以下是创建一个顺序表并实现插入和删除操作的基本步骤:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义顺序表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 初始化顺序表
Node* create_list() {
Node* head = (Node*)malloc(sizeof(Node));
if (!head) {
printf("Memory allocation failed.\n");
return NULL;
}
head->data = 0; // 初始为空
head->next = NULL;
return head;
}
// 插入元素到链表尾部
void insert(int value, Node** head_ref) {
Node* newNode = (Node*)malloc(sizeof(Node));
if (!newNode) {
printf("Memory allocation failed for new node.\n");
return;
}
newNode->data = value;
newNode->next = *head_ref;
*head_ref = newNode;
}
// 删除指定位置的元素
void delete_node(Node** head_ref, int position) {
if (*head_ref == NULL) {
printf("List is empty.\n");
return;
}
Node* temp = *head_ref;
Node* prev = NULL;
int count = 1;
// 遍历找到待删除的节点
while (temp != NULL && count < position) {
prev = temp;
temp = temp->next;
count++;
}
// 如果没找到对应位置,或者链表只有一个元素
if (count == position || temp == NULL) {
printf("Invalid position.\n");
return;
}
// 删除节点
prev->next = temp->next;
free(temp);
}
// 打印列表
void display(Node* head) {
Node* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
int main() {
Node* list = create_list();
// 插入元素示例
insert(10, &list);
insert(20, &list);
insert(30, &list);
// 显示列表
display(list);
// 删除元素示例
delete_node(&list, 1); // 删除索引为1的元素
// 再次显示列表
display(list);
return 0;
}
```
阅读全文
相关推荐
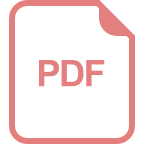
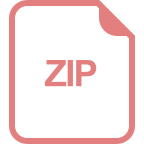
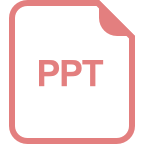





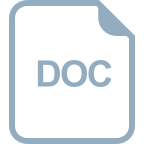









