输入10个数,首先对10个数进行排序并显示,然后输入1个数,通过折半查 找法查找该数在第几个数并显示,如果找不到则显示查无此数
时间: 2024-12-14 16:21:29 浏览: 3
这是一个简单的编程任务,通常在学习搜索算法时会被用来练习二分查找法。首先,你需要将用户输入的10个整数存储在一个数组中,然后对这个数组进行排序,常见的排序算法有冒泡排序、选择排序或快速排序等。例如,你可以使用C++的`std::sort()`函数。
排序后的数组,你可以通过二分查找法找到指定元素的位置。二分查找的工作原理是从中间开始,比较目标值与中间元素,如果目标值小,就在左半部分继续查找;如果大,则在右半部分查找,直到找到目标或确定目标不存在于数组中。C++的`std::binary_search()`函数可以简化这一过程。
下面是简单的伪代码示例:
```cpp
#include <iostream>
#include <algorithm>
void sortAndDisplay(int arr[], int size) {
std::sort(arr, arr + size);
for (int i = 0; i < size; ++i)
std::cout << arr[i] << " ";
std::cout << std::endl;
}
int binarySearch(int arr[], int target, int low, int high) {
if (low > high)
return -1;
int mid = low + (high - low) / 2;
if (arr[mid] == target)
return mid;
else if (arr[mid] < target)
return binarySearch(arr, target, mid + 1, high);
else
return binarySearch(arr, target, low, mid - 1);
}
int main() {
int nums[10];
// 读取并存储10个数
for (int i = 0; i < 10; ++i)
std::cin >> nums[i];
sortAndDisplay(nums, 10);
int searchNum;
std::cout << "Enter a number to search: ";
std::cin >> searchNum;
int position = binarySearch(nums, searchNum, 0, 9);
if (position != -1)
std::cout << "Number found at position: " << position + 1 << std::endl;
else
std::cout << "Number not found" << std::endl;
return 0;
}
```
阅读全文
相关推荐
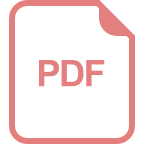
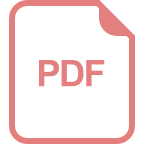
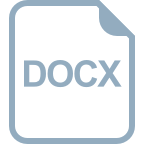













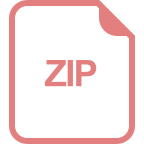
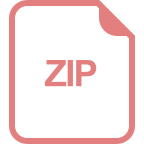
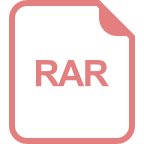