实现aes文件加密python算法
时间: 2024-10-15 18:19:19 浏览: 31
在Python中,你可以使用`cryptography`库来实现AES(Advanced Encryption Standard)文件加密。下面是一个简单的步骤示例:
首先,你需要安装`cryptography`库,可以使用pip来安装:
```bash
pip install cryptography
```
然后,你可以按照以下代码片段来进行文件的AES加密:
```python
from Crypto.Cipher import AES
from Crypto.Util.Padding import pad, unpad
import os
def encrypt_file(key, in_filename, out_filename):
# 加密模式选择,CBC需要一个随机IV
mode = AES.MODE_CBC
iv = os.urandom(16) # 生成16字节的随机IV
# 创建一个AES cipher对象,指定key和iv
cipher = AES.new(key, mode, iv)
# 打开输入文件,读取内容,并填充到AES块大小
with open(in_filename, 'rb') as infile:
content = infile.read()
content_padded = pad(content, AES.block_size)
# 对内容进行加密
ciphertext = cipher.encrypt(content_padded)
# 将IV和加密后的数据组合成一个新的二进制字符串
encrypted_data = iv + ciphertext
# 写入加密后的文件
with open(out_filename, 'wb') as outfile:
outfile.write(encrypted_data)
# 使用示例
key = b'sixteen_byte_key' # 16字节的密钥
in_filename = 'input.txt'
out_filename = 'output.enc'
encrypt_file(key, in_filename, out_filename)
```
对于解密操作,只需反向过程即可。注意实际应用中你需要妥善保存IV,因为它与加密数据一起提供给接收方用于解密。
阅读全文
相关推荐
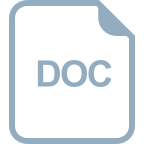
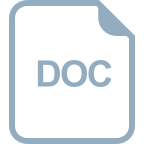
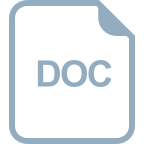









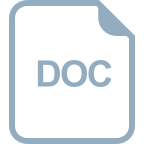
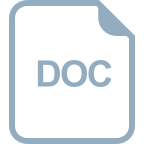
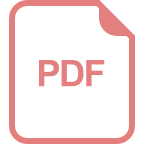
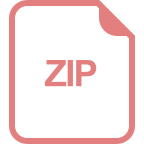
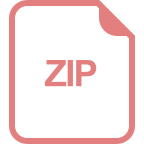
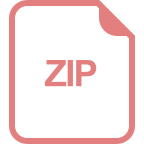
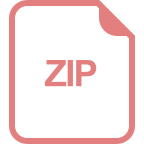
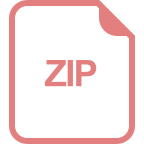