可以设置一个注意力机制(attention mechansim)来学习对应的权重向量Wc,该机制是从一个具有三个全连接层的DNN中获得的吗,python怎么实现
时间: 2024-09-18 16:05:29 浏览: 32
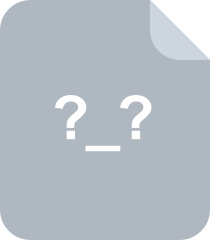
下面是一个自注意力机制(Self-Attention Mechanism)的实现,基于PyTorch
注意力机制(Attention Mechanism)是一种深度学习中的重要技术,用于处理序列数据,比如自然语言处理任务。它允许模型在处理输入时动态地赋予每个元素不同的权重,而不是简单地平均所有信息。这种机制通常不是直接从一个具有三个全连接层的DNN(密集连接神经网络)内生成的,而是作为单独的一个模块插入到DNN架构中。
在Python中,如使用TensorFlow或PyTorch等库,你可以这样实现:
1. TensorFlow:
```python
import tensorflow as tf
class AttentionLayer(tf.keras.layers.Layer):
def __init__(self, units, **kwargs):
super(AttentionLayer, self).__init__(**kwargs)
self.Wc = tf.Variable(tf.random.truncated_normal(shape=(units, 1)), name='weight_vector') # 初始化权重矩阵
self.dense_1 = tf.keras.layers.Dense(units, activation='relu')
self.dense_2 = tf.keras.layers.Dense(units)
def call(self, inputs, previous_attention_weights=None):
# 这里假设inputs是经过前几层处理后的张量
context_vector = self.dense_1(inputs)
attention_scores = tf.reduce_sum(context_vector * self.Wc, axis=1) # 计算得分
if previous_attention_weights is not None:
attention_scores += previous_attention_weights
attention_weights = tf.nn.softmax(attention_scores, axis=1) # 归一化
context_vector = tf.expand_dims(attention_weights, -1) * inputs # 加权求和
return context_vector, attention_weights
```
2. PyTorch:
```python
import torch
from torch import nn
class AttentionLayer(nn.Module):
def __init__(self, units):
super().__init__()
self.fc1 = nn.Linear(units, units)
self.fc2 = nn.Linear(units, 1)
self.Wc = nn.Parameter(torch.randn(1, units))
def forward(self, x, prev_attn_weights=None):
context = torch.relu(self.fc1(x))
scores = torch.matmul(context, self.Wc.t()) # 计算得分
if prev_attn_weights is not None:
scores += prev_attn_weights.unsqueeze(1)
attn_weights = F.softmax(scores, dim=1)
weighted_input = torch.bmm(attn_weights.unsqueeze(1), x.unsqueeze(2)).squeeze(1) # 加权求和
return weighted_input, attn_weights
```
在这两个例子中,我们创建了一个自定义的注意力层,包含全连接层和一个权重向量。在`forward`函数中,我们计算注意力分数并应用softmax归一化。
阅读全文
相关推荐
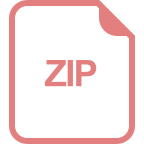
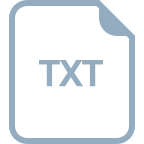
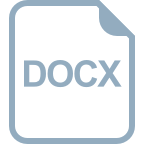
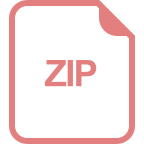
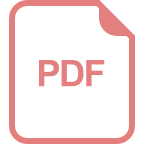
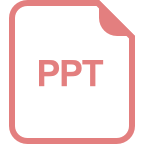
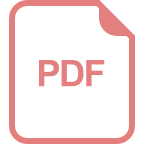
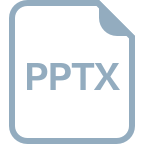
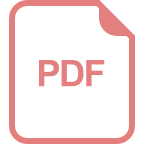
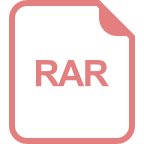
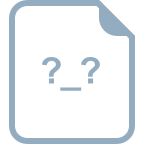
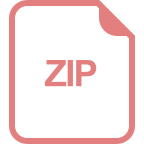
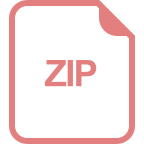
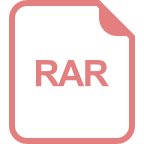
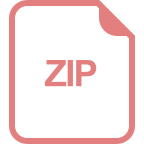
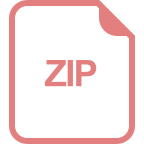