遗传算法python代码解释
时间: 2023-09-09 19:06:21 浏览: 42
遗传算法是一种基于生物进化原理的优化算法,常用于解决搜索和优化问题。以下是一个简单的遗传算法的Python代码示例,我会对其进行解释:
```python
import random
# 创建一个种群
def create_population(population_size, chromosome_size):
population = []
for _ in range(population_size):
chromosome = [random.randint(0, 1) for _ in range(chromosome_size)]
population.append(chromosome)
return population
# 计算适应度函数
def fitness_function(chromosome):
fitness = sum(chromosome) # 适应度函数为基因中1的个数
return fitness
# 选择操作
def selection(population):
# 使用轮盘赌选择策略
sum_fitness = sum([fitness_function(chromosome) for chromosome in population])
probabilities = [fitness_function(chromosome) / sum_fitness for chromosome in population]
selected_population = []
for _ in range(len(population)):
selected_chromosome = random.choices(population, probabilities)[0]
selected_population.append(selected_chromosome)
return selected_population
# 交叉操作
def crossover(population):
offspring_population = []
for i in range(0, len(population), 2):
parent1 = population[i]
parent2 = population[i+1]
# 选择一个交叉点
crossover_point = random.randint(1, len(parent1)-1)
# 进行交叉操作,生成两个子代
offspring1 = parent1[:crossover_point] + parent2[crossover_point:]
offspring2 = parent2[:crossover_point] + parent1[crossover_point:]
offspring_population.extend([offspring1, offspring2])
return offspring_population
# 变异操作
def mutation(population, mutation_rate):
for chromosome in population:
for i in range(len(chromosome)):
if random.random() < mutation_rate:
chromosome[i] = 1 - chromosome[i] # 突变操作:将0变为1,将1变为0
# 主函数
def genetic_algorithm(population_size, chromosome_size, num_generations, mutation_rate):
population = create_population(population_size, chromosome_size)
for _ in range(num_generations):
population = selection(population)
population = crossover(population)
mutation(population, mutation_rate)
best_chromosome = max(population, key=fitness_function)
best_fitness = fitness_function(best_chromosome)
return best_chromosome, best_fitness
# 使用示例
population_size = 100
chromosome_size = 10
num_generations = 50
mutation_rate = 0.01
best_chromosome, best_fitness = genetic_algorithm(population_size, chromosome_size, num_generations, mutation_rate)
print("Best Chromosome:", best_chromosome)
print("Best Fitness:", best_fitness)
```
上述代码是一个简单的遗传算法实现,包含了创建种群、计算适应度函数、选择、交叉和变异等基本操作。具体的解释如下:
1. `create_population` 函数用于创建一个种群,其中 `population_size` 参数表示种群中个体数量,`chromosome_size` 参数表示每个个体的染色体长度(基因个数)。
2. `fitness_function` 函数用于计算个体的适应度,这里简单地将适应度定义为染色体中1的个数。
3. `selection` 函数使用轮盘赌选择策略,根据个体的适应度进行选择操作,返回选择后的种群。
4. `crossover` 函数进行交叉操作,每次选择两个父代个体进行交叉,生成两个子代个体。
5. `mutation` 函数进行变异操作,根据给定的变异率对种群中的个体进行基因突变。
6. `genetic_algorithm` 函数是整个遗传算法的主函数,依次执行选择、交叉和变异操作,并返回最佳染色体和相应的适应度值。
7. 最后,我们通过调用 `genetic_algorithm` 函数得到最佳染色体和适应度,并输出结果。
这只是一个简单的遗传算法实现示例,实际使用中可以根据具体问题进行相应的修改和优化。
相关推荐
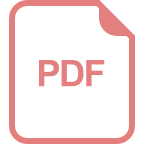










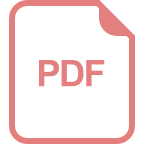