用Java写一个关于employee表的增删改查
时间: 2024-11-22 22:34:32 浏览: 11
在Java中,我们可以使用JDBC(Java Database Connectivity)来操作数据库,这里我将给出一个简单的Employee表的CRUD操作示例,假设我们有一个Employee表,包含id、name和email字段。
首先,你需要导入相关的包,并设置数据库连接:
```java
import java.sql.*;
public class EmployeeDAO {
private Connection connection;
// 构造函数获取数据库连接
public EmployeeDAO(Connection conn) {
this.connection = conn;
}
// 插入员工数据
public void insert(Employee employee) throws SQLException {
String sql = "INSERT INTO employee (id, name, email) VALUES (?, ?, ?)";
try (PreparedStatement pstmt = connection.prepareStatement(sql)) {
pstmt.setInt(1, employee.getId());
pstmt.setString(2, employee.getName());
pstmt.setString(3, employee.getEmail());
pstmt.executeUpdate();
}
}
// 更新员工信息
public void update(Employee employee) throws SQLException {
String sql = "UPDATE employee SET name = ?, email = ? WHERE id = ?";
try (PreparedStatement pstmt = connection.prepareStatement(sql)) {
pstmt.setString(1, employee.getName());
pstmt.setString(2, employee.getEmail());
pstmt.setInt(3, employee.getId());
pstmt.executeUpdate();
}
}
// 删除员工
public void delete(int id) throws SQLException {
String sql = "DELETE FROM employee WHERE id = ?";
try (PreparedStatement pstmt = connection.prepareStatement(sql)) {
pstmt.setInt(1, id);
pstmt.executeUpdate();
}
}
// 查询所有员工
public List<Employee> getAll() throws SQLException {
List<Employee> employees = new ArrayList<>();
String sql = "SELECT * FROM employee";
try (Statement stmt = connection.createStatement();
ResultSet rs = stmt.executeQuery(sql)) {
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
String email = rs.getString("email");
employees.add(new Employee(id, name, email));
}
}
return employees;
}
}
```
这个类中,每个方法对应着数据库操作的SQL语句。注意这只是一个基本示例,实际应用中还需要处理异常并添加事务控制。
阅读全文
相关推荐
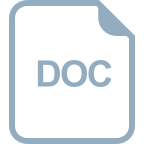
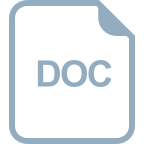
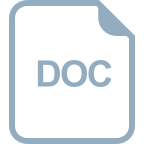
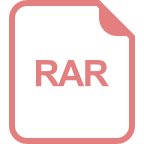


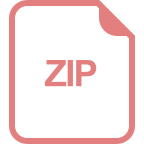
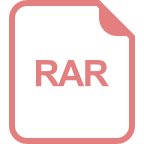
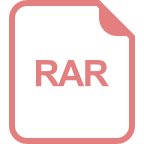
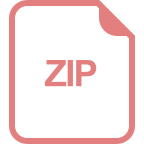
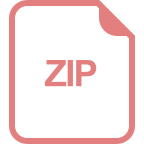
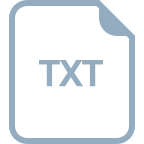
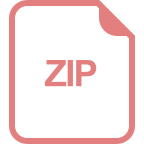





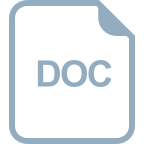