编写一个 Python 脚本,读取一个目录中的所有文本文件(假设文件扩展名为 `.txt`),并统计每个文件中单词的总数。将结果保存到一个名为 `word_count.txt` 的文件中,格式如下
时间: 2024-11-25 11:11:54 浏览: 14
编写一个Python脚本来完成这个任务,可以使用os模块来遍历目录,`re`模块来处理文本内容并提取单词,最后将结果写入新的文件。以下是一个简单的示例:
```python
import os
import re
# 定义函数来读取并统计单词数
def count_words_in_file(file_path):
with open(file_path, 'r', encoding='utf-8') as f:
text = f.read()
words = re.findall(r'\b\w+\b', text) # 使用正则表达式找到所有的单词
return len(words)
# 目录路径
directory_path = 'path/to/your/directory'
# 所有.txt文件列表
text_files = [f for f in os.listdir(directory_path) if f.endswith('.txt')]
# 初始化总词数
total_word_count = 0
# 统计每个文件的单词数并累加
for file_name in text_files:
file_path = os.path.join(directory_path, file_name)
word_count = count_words_in_file(file_path)
total_word_count += word_count
print(f"{file_name}: {word_count} words")
# 将结果写入'word_count.txt'
with open('word_count.txt', 'w', encoding='utf-8') as output_file:
output_file.write(f"Total words across all files: {total_word_count}\n")
for file_name, word_count in zip(text_files, word_count_list): # 假设我们之前保存了word_count_list
output_file.write(f"{file_name}: {word_count}\n")
阅读全文
相关推荐
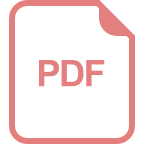
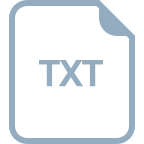
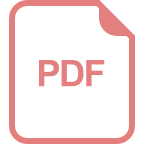



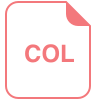
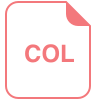
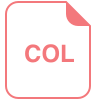
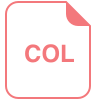
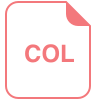
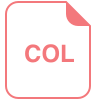
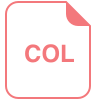
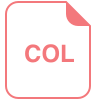
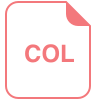
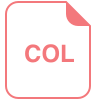
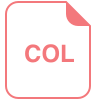
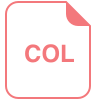
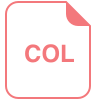