编写一个python脚本,读取一个目录中的所有文本文件(假设文件扩展名为'.txt'),并统计每个文件中单词的总数。将结果保存到一个名为'word_count.txt'的文件格式中,格式如下:filename1.txt:123words
时间: 2024-11-22 17:41:34 浏览: 20
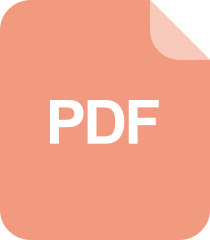
Python读取一个目录下所有目录和文件的方法

你可以创建一个Python脚本来遍历指定目录下的所有`.txt`文件,并分别统计每个文件中的单词数。这里是一个示例脚本:
```python
import os
import re
from collections import defaultdict
def count_words_in_file(file_path):
with open(file_path, 'r', encoding='utf-8') as file:
text = file.read()
words = re.findall(r'\b\w+\b', text.lower()) # 使用正则表达式提取单词并转为小写
return len(words)
def process_directory(directory, output_file):
word_count_dict = defaultdict(int)
for filename in os.listdir(directory):
if filename.endswith('.txt'):
file_path = os.path.join(directory, filename)
word_count_dict[filename] += count_words_in_file(file_path)
with open(output_file, 'w', newline='', encoding='utf-8') as out_file:
for filename, word_count in word_count_dict.items():
out_file.write(f"{filename}.txt:{word_count}words\n")
# 调用函数,给定一个目录路径和输出文件名
process_directory('/path/to/your/directory', 'word_count.txt')
```
在这个脚本中,我们首先导入所需的库,然后定义两个函数:`count_words_in_file`用于统计单个文件内的单词数,`process_directory`用于遍历目录并生成最终的输出文件。
阅读全文
相关推荐
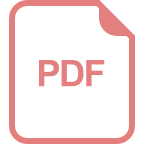
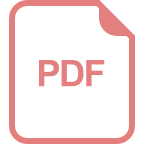


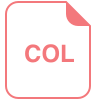
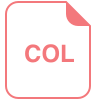
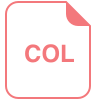
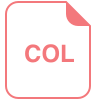
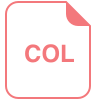
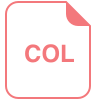
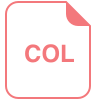
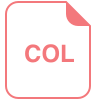
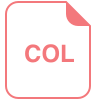
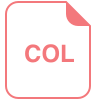
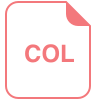
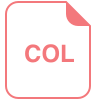
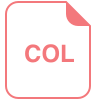
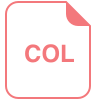