数据结构(c语言版)中写利用折半查找法查找员工信息。要求:创建某公司员工信息表,表中记录员工的ID,姓名,性别和电话,表中记录按照id号递增有序,利用折半查找快速查找指定20号的员工的代码使用VC6.0
时间: 2024-12-19 15:19:43 浏览: 21
在C语言中,你可以使用二分查找算法(折半查找法)来查找特定编号(例如20号)的员工。首先,你需要定义一个结构体来表示员工信息,并创建一个动态数组来存储所有员工。下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义员工信息结构体
typedef struct {
int id;
char name[50];
char gender[10];
char phone[20];
} Employee;
// 助手函数,用于比较两个员工的ID
int compare(const void* a, const void* b) {
Employee *empA = (Employee*)a;
Employee *empB = (Employee*)b;
return empA->id - empB->id;
}
// 折半查找函数
Employee* binary_search(int arr[], int n, int target_id) {
int low = 0, high = n - 1;
while (low <= high) {
int mid = low + (high - low) / 2;
if (arr[mid].id == target_id)
return &arr[mid]; // 找到目标员工,返回指针
else if (arr[mid].id < target_id)
low = mid + 1; // 目标ID大于中间元素,缩小左半部分搜索范围
else
high = mid - 1; // 目标ID小于中间元素,缩小右半部分搜索范围
}
// 没找到,返回NULL
return NULL;
}
int main() {
Employee employees[] = {
// 假设这里已经填充了按ID升序排列的员工信息...
};
int num_employees = sizeof(employees) / sizeof(employees[0]);
Employee* result = binary_search(employees, num_employees, 20);
if (result != NULL) {
printf("找到员工信息:\n");
printf("ID: %d\n", result->id);
printf("姓名: %s\n", result->name);
printf("性别: %s\n", result->gender);
printf("电话: %s\n", result->phone);
} else {
printf("未找到ID为20的员工。\n");
}
return 0;
}
```
在这个例子中,`binary_search`函数接收一个排序好的员工数组、数组长度以及要查找的目标ID。它会在每次迭代中将搜索区间减半,直到找到目标员工或者搜索区间为空。
阅读全文
相关推荐
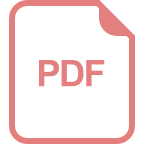
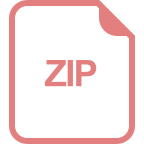
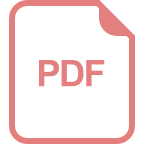















