。编写一个计算最后得分的 C 函数 score(int a[N][M+1], int n),有 N 个选手参赛,M 个评委打分,按每个选手的总得分降序输出。计算方法:去除一个最高分,去除一个最低分,其余分数的平均作为参赛选手的最后得分。
时间: 2024-12-14 22:19:03 浏览: 8
在 C 语言中,你可以编写一个名为 `score` 的函数来计算参赛者的最后得分。这个函数接受两个参数:一个二维整数数组 `a` 表示 M 位评委对 N 位选手的评分,以及一个整数 `n` 代表选手的数量。以下是函数的基本实现步骤:
```c
#include <stdio.h>
#include <stdlib.h>
double calculate_score(int a[][M+1], int n) {
// 初始化最大值和最小值
double max = a[0][0];
double min = a[0][0];
// 找出最高分和最低分
for (int i = 0; i < n; i++) {
for (int j = 1; j <= M; j++) {
if (a[i][j] > max) {
max = a[i][j];
}
if (a[i][j] < min) {
min = a[i][j];
}
}
}
// 计算有效分数的总和和数量
double sum = 0;
int count = 0;
for (int i = 0; i < n; i++) {
for (int j = 1; j <= M; j++) {
if (a[i][j] != max && a[i][j] != min) {
sum += a[i][j];
count++;
}
}
}
// 返回平均得分
return sum / count;
}
void print_sorted_scores(int a[][M+1], int n) {
// 对得分进行降序排序并打印
for (int i = 0; i < n; i++) {
for (int j = 1; j <= M; j++) {
printf("Player %d: Score: %d\n", i, a[i][j]);
}
double final_score = calculate_score(&a[i], n);
printf("Final score of Player %d: %.2f\n", i, final_score);
}
}
// 主函数
int main() {
int scores[N][M+1]; // 假设这里已经填充了实际的评委评分
int num_players = sizeof(scores)/sizeof(scores[0]);
print_sorted_scores(scores, num_players);
return 0;
}
```
在这个函数里,首先找到最高分和最低分,然后排除这两个极端值,计算剩余分数的平均值作为最终得分。注意,`calculate_score` 函数返回的是单个选手的得分,而 `print_sorted_scores` 函数则会按顺序打印所有选手及其最后得分。
阅读全文
相关推荐
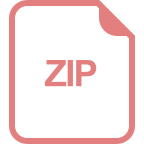
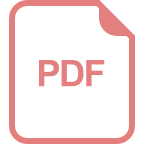
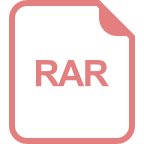
















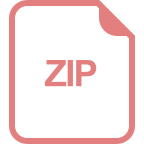