2. (简答题) 编写一个二维数组类double A[m][n],通过函数计算该数组的最大值、最小值、均值,一并返回并打印输出
时间: 2024-12-23 20:27:41 浏览: 7
你可以创建一个名为`DoubleMatrix`的类,其中包含一个二维双精度数组,并提供相应的成员函数来计算最大值、最小值和均值。以下是简单的实现:
```cpp
#include <iostream>
#include <vector>
class DoubleMatrix {
private:
std::vector<std::vector<double>> matrix; // 二维数组存储
int m, n;
public:
DoubleMatrix(int rows, int cols) : m(rows), n(cols) {} // 构造函数
void setValues(const std::vector<std::vector<double>>& values) { // 设置矩阵值
matrix = values;
}
double getMax() const { // 获取最大值
double max = matrix[0][0];
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
if (matrix[i][j] > max)
max = matrix[i][j];
}
}
return max;
}
double getMin() const { // 获取最小值
double min = matrix[0][0];
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
if (matrix[i][j] < min)
min = matrix[i][j];
}
}
return min;
}
double getMean() const { // 计算均值
double sum = 0.0;
for (int i = 0; i < m; i++) {
for (int j = 0; j < n; j++) {
sum += matrix[i][j];
}
}
return sum / (m * n);
}
void printMatrix() const { // 打印矩阵
for (const auto& row : matrix) {
for (double val : row) {
std::cout << val << " ";
}
std::cout << "\n";
}
}
void calculateAndPrintStats() const { // 同时计算并打印统计值
double max_val = getMax();
double min_val = getMin();
double mean_val = getMean();
std::cout << "最大值: " << max_val << "\n";
std::cout << "最小值: " << min_val << "\n";
std::cout << "均值: " << mean_val << "\n";
printMatrix();
}
};
int main() {
int m = 4, n = 5;
DoubleMatrix mat(m, n);
// 例如设置矩阵的值
std::vector<std::vector<double>> values = {{1, 2, 3, 4, 5},
{6, 7, 8, 9, 10},
{11, 12, 13, 14, 15},
{16, 17, 18, 19, 20}};
mat.setValues(values);
mat.calculateAndPrintStats(); // 调用计算并打印
return 0;
}
```
在这个例子中,`calculateAndPrintStats`函数会同时计算并打印出二维数组的统计信息以及整个矩阵内容。
阅读全文
相关推荐
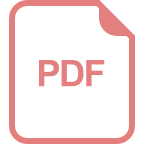















