python rsa解密
时间: 2024-10-23 09:20:44 浏览: 17
要使用Python RSA库进行解密,首先确保已安装`pycryptodome`(它是Python RSA的一个替代库),因为原版的`Python_RSA`已被弃用。下面是如何使用`pycryptodome`进行RSA解密的基本示例:
```python
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
# 假设你有一个公钥(pem格式)和对应的私钥
public_key = load_pem_publickey('path_to_public.pem')
private_key = load_pem_privatekey('path_to_private.pem')
# 加载待解密的数据(以十六进制表示)
ciphertext = "your_encrypted_data_in_hex"
# 使用私钥解密
cipher_rsa = PKCS1_OAEP.new(private_key)
plaintext = cipher_rsa.decrypt(ciphertext.encode())
print(f"Decrypted message: {plaintext.decode()}") # 解密后的文本
```
在这个例子中,你需要替换`load_pem_publickey`和`load_pem_privatekey`函数的参数,以及`your_encrypted_data_in_hex`为你实际的加密数据。
相关问题
python rsa 解密
RSA是一种非对称加密算法,在Python中可以使用pycryptodome库来进行RSA的加密和解密操作。具体的使用方法如下:
1. 安装pycryptodome库: `pip install pycryptodome`
2. 生成RSA密钥对:
```
from Crypto.PublicKey import RSA
# 生成密钥对
key = RSA.generate(2048)
# 保存公钥
with open('public.pem', 'wb') as f:
f.write(key.publickey().export_key())
# 保存私钥
with open('private.pem', 'wb') as f:
f.write(key.export_key())
```
3. 加密:
```
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
# 从文件中读取公钥
with open('public.pem', 'rb') as f:
key = RSA.import_key(f.read())
# 初始化加密器
cipher = PKCS1_OAEP.new(key)
# 需要加密的明文
plaintext = b'my plaintext'
# 加密
ciphertext = cipher.encrypt(plaintext)
```
4. 解密:
```
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
# 从文件中读取私钥
with open('private.pem', 'rb') as f:
key = RSA.import_key(f.read())
# 初始化解密器
cipher = PKCS1_OAEP.new(key)
# 解密
plaintext = cipher.decrypt(ciphertext)
```
希望这个能帮到你!
python rsa解密需要什么
在Python中使用rsa库进行解密时,你需要以下几个关键组件[^1]:
1. 导入必要的模块:
```python
import rsa
from base64 import b64decode
```
2. 拥有私钥(PrivateKey)用于解密,这是RSA算法中的核心部分。如果你已经有了密钥对,可以这样加载私钥:
```python
with open('private_key.pem', 'rb') as f:
private_key = rsa.PrivateKey.load_pkcs1(f.read())
```
注意这里的`private_key.pem`是你存储私钥文件的实际路径。
3. 使用`decrypt`函数进行解密,输入是Base64编码的数据:
```python
encoded_data = 'base64_encoded_string' # 替换为你实际接收到的Base64编码的密文
decoded_data = b64decode(encoded_data)
decrypted_text = rsa.decrypt(decoded_data, private_key)
```
如果`None`作为第二个参数传递(如`cipher_rsa.decrypt(base64_data, None)`),它表示默认使用PKCS#1 padding(如果数据不满足标准长度可能会抛出异常,你可以选择捕获并处理这个错误,比如用`DecryptError`替换)。
阅读全文
相关推荐
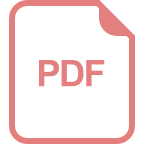
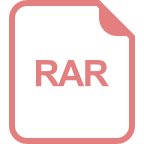
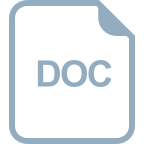









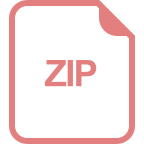


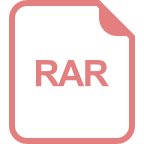