进程调度算法 Python
时间: 2024-09-28 09:00:20 浏览: 37
进程调度算法是一种操作系统管理任务执行顺序的方式,它决定了哪些进程何时以及如何从就绪队列中获取CPU资源进行执行。在Python中,虽然直接操作进程调度不是语言的一部分,但我们可以通过一些库如`multiprocessing`或`concurrent.futures`间接应用调度策略。
常见的进程调度算法有:
1. **先来先服务** (FCFS,First-Come, First-Served):按照进程到达的时间顺序进行处理。
2. **短作业优先** (SJF,Shortest Job First):优先执行剩余时间最短的任务。
3. **优先级调度**:每个进程分配一个优先级,高优先级的进程先执行。
4. **时间片轮转** (Round Robin, RR):按固定时间片轮流给每个进程分配CPU时间。
5. **抢占式优先级调度**:类似RR,但允许高优先级进程中断低优先级进程。
在Python中,你可以通过`ThreadPoolExecutor`或`ProcessPoolExecutor`来自行设置线程池或进程池的工作队列策略,这在一定程度上模拟了调度算法的行为。例如,可以设置`max_workers`来控制并发数,或者通过`ThreadPoolExecutor.map()`和`apply_async()`的`chunksize`参数调整任务分片,间接实现了某种形式的调度。
相关问题
进程调度算法python实现
进程调度算法是指操作系统如何决定哪个进程应该获得处理器的时间片,常见的调度算法有先来先服务(FCFS)、短作业优先(SJF)、优先级调度、循环轮转法等。在Python中,虽然核心操作系统级别的调度由内核处理,但我们可以通过模拟简单的线程调度来理解算法。
例如,如果你想要实现一个简单版本的基于时间片的抢占式调度,你可以使用`threading`模块创建线程,并维护一个队列来存储待调度的任务。下面是一个简单的示例:
```python
import threading
import time
class Process:
def __init__(self, name, priority):
self.name = name
self.priority = priority
self.start_time = time.time()
class Scheduler:
def __init__(self, quantum=0.5):
self.quantum = quantum
self.queue = []
self.current_process = None
def schedule(self):
if not self.queue:
return
self.current_process = self.queue.pop(0)
print(f'Starting {self.current_process.name} with priority {self.current_process.priority}')
time.sleep(self.current_process.priority * self.quantum) # 模拟进程运行时间
print(f'{self.current_process.name} finished in {time.time() - self.current_process.start_time} seconds')
# 创建并添加进程到调度器
scheduler = Scheduler()
scheduler.queue.extend([Process('Task1', 0.3), Process('Task2', 0.8), Process('Task3', 0.1)])
# 开始调度
while scheduler.queue:
scheduler.schedule()
```
这个例子展示了基本的抢占式调度,每次从队列中取出优先级最高的进程开始执行,直到其执行完一个时间片。
多级反馈队列进程调度算法python
多级反馈队列(Multi-Level Feedback Queue)是一种进程调度算法,它将进程划分为多个队列,每个队列有不同的优先级。当一个进程到达系统时,它首先被放入最高优先级的队列中运行,如果它在时间片内完成任务,则进入下一轮的当前队列,如果没有完成任务,则被移到下一级队列中等待下一次调度。这样,根据进程的行为和执行时间,可以动态地调整进程的优先级。
下面是一个用Python实现多级反馈队列进程调度算法的示例:
```python
class Process:
def __init__(self, pid, burst_time):
self.pid = pid
self.burst_time = burst_time
self.remaining_time = burst_time
def multi_level_feedback_queue(processes, time_quantum):
queues = [[] for _ in range(3)] # 三个优先级队列
queue_idx = 0 # 当前队列索引
for process in processes:
queues[0].append(process) # 将所有进程放入最高优先级队列
while True:
if not any(queues): # 所有队列都为空时退出循环
break
if queue_idx >= len(queues):
queue_idx = 0
if not queues[queue_idx]: # 当前队列为空时跳过
queue_idx += 1
continue
current_process = queues[queue_idx].pop(0)
if current_process.remaining_time <= time_quantum:
print(f"Process {current_process.pid} completed.")
else:
current_process.remaining_time -= time_quantum
print(f"Process {current_process.pid} timed out. Remaining time: {current_process.remaining_time}")
queues[queue_idx + 1].append(current_process)
queue_idx += 1
# 测试示例
processes = [Process(1, 10), Process(2, 5), Process(3, 8)]
time_quantum = 4
multi_level_feedback_queue(processes, time_quantum)
```
阅读全文
相关推荐
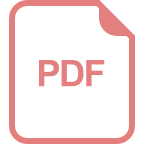
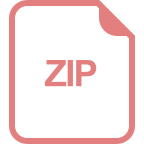







