C语言推箱子代码详细注释
时间: 2024-12-15 10:20:38 浏览: 40
C语言编写推箱子游戏的代码通常涉及到基本的游戏逻辑、数据结构和函数设计。由于这里是一个文本环境,我会提供一个简化版的示例并给出关键部分的注释,实际游戏开发会更复杂,涉及图形界面和更多的用户交互。
```c
#include <stdio.h>
#include <stdlib.h> // 引入内存管理头文件
// 定义棋盘大小和箱子位置结构体
#define BOARD_SIZE 4
typedef struct {
int x; // 箱子横坐标
int y; // 箱子纵坐标
} Box;
// 定义棋盘和箱子数组
Box board[BOARD_SIZE][BOARD_SIZE] = {0};
int has_box[BOARD_SIZE][BOARD_SIZE]; // 存储是否有箱子的位置
// 初始化棋盘,开始时所有格子都没有箱子
void init_board() {
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
if (i == 0 || j == 0 || i == BOARD_SIZE - 1 || j == BOARD_SIZE - 1) {
board[i][j].x = j;
board[i][j].y = i;
has_box[i][j] = 1;
} else {
board[i][j].x = j;
board[i][j].y = i;
has_box[i][j] = 0;
}
}
}
}
// 检查玩家能否移动到目标位置
int can_move(int dx, int dy) {
int newX = board[current_player_x][current_player_y].x + dx;
int newY = board[current_player_x][current_player_y].y + dy;
// 玩家不能走出棋盘范围
if (newX < 0 || newX >= BOARD_SIZE || newY < 0 || newY >= BOARD_SIZE)
return 0;
// 玩家不能把箱子放在墙上
if (!has_box[newX][newY])
return 1;
return 0;
}
// 主游戏循环
void main_game_loop() {
printf("请输入方向键(WASD):");
// ...此处省略处理用户输入和移动逻辑...
}
int main() {
init_board();
current_player_x = 0; // 设定初始玩家位置
current_player_y = BOARD_SIZE - 1;
main_game_loop();
return 0;
}
```
阅读全文
相关推荐
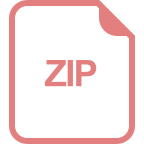
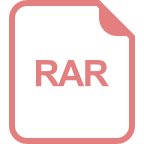
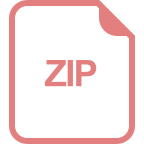
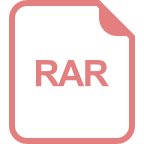
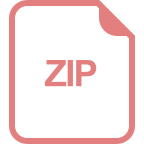
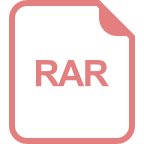
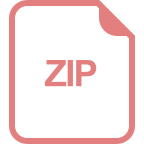
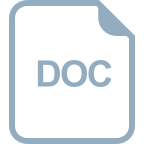
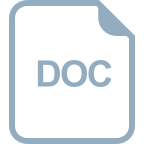
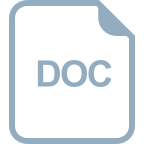
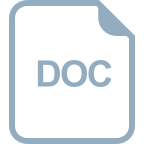
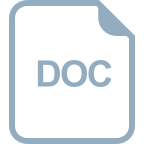
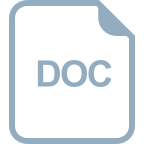
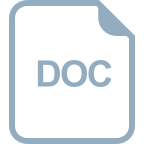
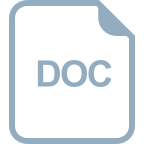



