牛顿迭代法求根matlab
时间: 2023-11-05 20:05:43 浏览: 301
在Matlab中,可以使用以下代码实现牛顿迭代法求根:
假设要求解方程 f(x) = 0 的根,初始值为 x0,迭代公式为 x(i+1) = x(i) - f(x(i))/f'(x(i)),其中 f'(x) 表示 f(x) 的导数。
代码如下:
```matlab
syms x; % 定义符号变量 x
f = x^3 - 2*x - 5; % 定义方程 f(x)
df = diff(f); % 求导数 f'(x)
x0 = 2; % 初始值
tol = 1e-6; % 精度要求
max_iter = 100; % 最大迭代次数
for i = 1:max_iter
fx0 = double(subs(f, x, x0)); % 计算 f(x0)
dfx0 = double(subs(df, x, x0)); % 计算 f'(x0)
x1 = x0 - fx0/dfx0; % 计算下一个迭代点 x1
if abs(x1 - x0) < tol % 判断是否满足精度要求
break;
end
x0 = x1; % 更新迭代点
end
disp(['方程的根为:', num2str(x1)]); % 输出结果
```
相关问题
牛顿迭代法求根matlab程序
牛顿迭代法是一种用于求解方程根的迭代方法,它通过不断逼近函数的根来得到精确的解。下面是一个用MATLAB实现牛顿迭代法求根的程序示例:
```matlab
function [root, iterations] = newtonMethod(f, df, x0, epsilon, maxIterations)
% f: 待求根的函数
% df: f的导数函数
% x0: 初始猜测值
% epsilon: 精度要求
% maxIterations: 最大迭代次数
iterations = 0;
while abs(f(x0)) > epsilon && iterations < maxIterations
x0 = x0 - f(x0) / df(x0);
iterations = iterations + 1;
end
root = x0;
end
```
使用该程序,你需要提供以下参数:
- `f`:待求根的函数,可以是一个匿名函数或者函数句柄。
- `df`:`f`的导数函数,同样可以是一个匿名函数或者函数句柄。
- `x0`:初始猜测值。
- `epsilon`:精度要求,当函数值的绝对值小于该值时,认为已经找到了根。
- `maxIterations`:最大迭代次数,防止无限循环。
程序会返回两个结果:
- `root`:求得的根。
- `iterations`:实际迭代次数。
请注意,使用牛顿迭代法求根时,初始猜测值的选择对结果的精度和收敛速度有很大影响。如果初始猜测值选择不当,可能会导致迭代过程发散或者收敛到错误的根。因此,在使用牛顿迭代法时,需要根据具体问题选择合适的初始猜测值。
牛顿迭代法求函数matlab
牛顿迭代法是一种用于寻找方程的根的迭代方法,其基本思想是利用泰勒展开式的前若干项来逼近函数的零点。具体来说,牛顿迭代法通过不断逼近函数的零点来求解方程,每一次迭代都会利用当前估计值的导数来更新估计值,直到达到预设的精度或者迭代次数。在 MATLAB 中,可以使用 fzero 函数来实现牛顿迭代法求解方程的功能。具体使用方法如下:
1. 定义需要求解的方程,例如 x^3 - 2x - 5 = 0
2. 调用 fzero 函数,将方程和一个初始估计值作为参数传入,例如 fzero(@(x) x^3 - 2*x - 5, 1)
3. fzero 函数将返回方程的根
阅读全文
相关推荐
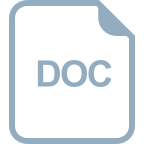
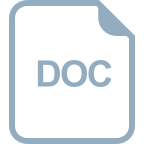
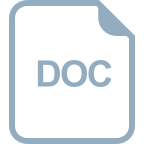
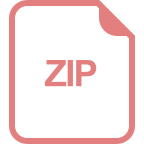
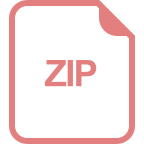
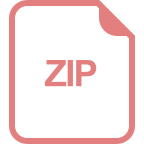

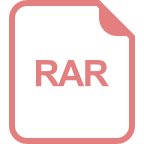
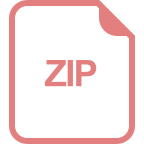
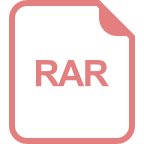





